Категорії
Електронні книги
-
Бізнес та економіка
- Біткойн
- Ділова жінка
- Коучинг
- Контроль
- Електронний бізнес
- Економіка
- Фінанси
- Фондова біржа та інвестиції
- Особисті компетенції
- Комп'ютер в офісі
- Комунікація та переговори
- Малий бізнес
- Маркетинг
- Мотивація
- Мультимедійне навчання
- Нерухомість
- Переконання та НЛП
- Податки
- Соціальна політика
- Порадники
- Презентації
- Лідерство
- Зв'язки з громадськістю
- Звіти, аналізи
- Секрет
- Соціальні засоби комунікації
- Продаж
- Стартап
- Ваша кар'єра
- Управління
- Управління проектами
- Людські ресурси (HR)
-
Для дітей
-
Для молоді
-
Освіта
-
Енциклопедії, словники
-
Електронна преса
- Architektura i wnętrza
- Безпека життєдіяльності
- Biznes i Ekonomia
- Будинок та сад
- Електронний бізнес
- Ekonomia i finanse
- Езотерика
- Фінанси
- Особисті фінанси
- Бізнес
- Фотографія
- Інформатика
- Відділ кадрів та оплата праці
- Для жінок
- Комп'ютери, Excel
- Бухгалтерія
- Культура та література
- Наукові та академічні
- Охорона навколишнього середовища
- Впливові
- Освіта
- Податки
- Подорожі
- Психологія
- Релігія
- Сільське господарство
- Ринок книг і преси
- Транспорт та спедиція
- Здоров'я та краса
-
Історія
-
Інформатика
- Офісні застосунки
- Бази даних
- Біоінформатика
- Бізнес ІТ
- CAD/CAM
- Digital Lifestyle
- DTP
- Електроніка
- Цифрова фотографія
- Комп'ютерна графіка
- Ігри
- Хакування
- Hardware
- IT w ekonomii
- Наукові пакети
- Шкільні підручники
- Основи комп'ютера
- Програмування
- Мобільне програмування
- Інтернет-сервери
- Комп'ютерні мережі
- Стартап
- Операційні системи
- Штучний інтелект
- Технологія для дітей
- Вебмайстерність
-
Інше
-
Іноземні мови
-
Культура та мистецтво
-
Шкільні читанки
-
Література
- Антології
- Балада
- Біографії та автобіографії
- Для дорослих
- Драми
- Журнали, щоденники, листи
- Епос, епопея
- Нарис
- Наукова фантастика та фантастика
- Фельєтони
- Художня література
- Гумор, сатира
- Інше
- Класичний
- Кримінальний роман
- Нехудожня література
- Художня література
- Mity i legendy
- Лауреати Нобелівської премії
- Новели
- Побутовий роман
- Okultyzm i magia
- Оповідання
- Спогади
- Подорожі
- Оповідна поезія
- Поезія
- Політика
- Науково-популярна
- Роман
- Історичний роман
- Проза
- Пригодницька
- Журналістика
- Роман-репортаж
- Romans i literatura obyczajowa
- Сенсація
- Трилер, жах
- Інтерв'ю та спогади
-
Природничі науки
-
Соціальні науки
-
Шкільні підручники
-
Науково-популярна та академічна
- Археологія
- Bibliotekoznawstwo
- Кінознавство / Теорія кіно
- Філологія
- Польська філологія
- Філософія
- Finanse i bankowość
- Географія
- Економіка
- Торгівля. Світова економіка
- Історія та археологія
- Історія мистецтва і архітектури
- Культурологія
- Мовознавство
- літературні студії
- Логістика
- Математика
- Ліки
- Гуманітарні науки
- Педагогіка
- Навчальні засоби
- Науково-популярна
- Інше
- Психологія
- Соціологія
- Театральні студії
- Богослов’я
- Економічні теорії та науки
- Transport i spedycja
- Фізичне виховання
- Zarządzanie i marketing
-
Порадники
-
Ігрові посібники
-
Професійні та спеціальні порадники
-
Юридична
- Безпека життєдіяльності
- Історія
- Дорожній кодекс. Водійські права
- Юридичні науки
- Охорона здоров'я
- Загальне, компендіум
- Академічні підручники
- Інше
- Закон про будівництво і житло
- Цивільне право
- Фінансове право
- Господарське право
- Господарське та комерційне право
- Кримінальний закон
- Кримінальне право. Кримінальні злочини. Кримінологія
- Міжнародне право
- Міжнародне та іноземне право
- Закон про охорону здоров'я
- Закон про освіту
- Податкове право
- Трудове право та законодавство про соціальне забезпечення
- Громадське, конституційне та адміністративне право
- Кодекс про шлюб і сім'ю
- Аграрне право
- Соціальне право, трудове право
- Законодавство Євросоюзу
- Промисловість
- Сільське господарство та захист навколишнього середовища
- Словники та енциклопедії
- Державні закупівлі
- Управління
-
Путівники та подорожі
- Африка
- Альбоми
- Південна Америка
- Центральна та Північна Америка
- Австралія, Нова Зеландія, Океанія
- Австрія
- Азії
- Балкани
- Близький Схід
- Болгарія
- Китай
- Хорватія
- Чеська Республіка
- Данія
- Єгипет
- Естонія
- Європа
- Франція
- Гори
- Греція
- Іспанія
- Нідерланди
- Ісландія
- Литва
- Латвія
- Mapy, Plany miast, Atlasy
- Мініпутівники
- Німеччина
- Норвегія
- Активні подорожі
- Польща
- Португалія
- Інше
- Przewodniki po hotelach i restauracjach
- Росія
- Румунія
- Словаччина
- Словенія
- Швейцарія
- Швеція
- Світ
- Туреччина
- Україна
- Угорщина
- Велика Британія
- Італія
-
Психологія
- Філософія життя
- Kompetencje psychospołeczne
- Міжособистісне спілкування
- Mindfulness
- Загальне
- Переконання та НЛП
- Академічна психологія
- Психологія душі та розуму
- Психологія праці
- Relacje i związki
- Батьківство та дитяча психологія
- Вирішення проблем
- Інтелектуальний розвиток
- Секрет
- Сексуальність
- Спокушання
- Зовнішній вигляд та імідж
- Філософія життя
-
Релігія
-
Спорт, фітнес, дієти
-
Техніка і механіка
Аудіокниги
-
Бізнес та економіка
- Біткойн
- Ділова жінка
- Коучинг
- Контроль
- Електронний бізнес
- Економіка
- Фінанси
- Фондова біржа та інвестиції
- Особисті компетенції
- Комунікація та переговори
- Малий бізнес
- Маркетинг
- Мотивація
- Нерухомість
- Переконання та НЛП
- Податки
- Соціальна політика
- Порадники
- Презентації
- Лідерство
- Зв'язки з громадськістю
- Секрет
- Соціальні засоби комунікації
- Продаж
- Стартап
- Ваша кар'єра
- Управління
- Управління проектами
- Людські ресурси (HR)
-
Для дітей
-
Для молоді
-
Освіта
-
Енциклопедії, словники
-
Електронна преса
-
Історія
-
Інформатика
-
Інше
-
Іноземні мови
-
Культура та мистецтво
-
Шкільні читанки
-
Література
- Антології
- Балада
- Біографії та автобіографії
- Для дорослих
- Драми
- Журнали, щоденники, листи
- Епос, епопея
- Нарис
- Наукова фантастика та фантастика
- Фельєтони
- Художня література
- Гумор, сатира
- Інше
- Класичний
- Кримінальний роман
- Нехудожня література
- Художня література
- Mity i legendy
- Лауреати Нобелівської премії
- Новели
- Побутовий роман
- Okultyzm i magia
- Оповідання
- Спогади
- Подорожі
- Поезія
- Політика
- Науково-популярна
- Роман
- Історичний роман
- Проза
- Пригодницька
- Журналістика
- Роман-репортаж
- Romans i literatura obyczajowa
- Сенсація
- Трилер, жах
- Інтерв'ю та спогади
-
Природничі науки
-
Соціальні науки
-
Науково-популярна та академічна
-
Порадники
-
Професійні та спеціальні порадники
-
Юридична
-
Путівники та подорожі
-
Психологія
- Філософія життя
- Міжособистісне спілкування
- Mindfulness
- Загальне
- Переконання та НЛП
- Академічна психологія
- Психологія душі та розуму
- Психологія праці
- Relacje i związki
- Батьківство та дитяча психологія
- Вирішення проблем
- Інтелектуальний розвиток
- Секрет
- Сексуальність
- Спокушання
- Зовнішній вигляд та імідж
- Філософія життя
-
Релігія
-
Спорт, фітнес, дієти
-
Техніка і механіка
Відеокурси
-
Бази даних
-
Big Data
-
Biznes, ekonomia i marketing
-
Кібербезпека
-
Data Science
-
DevOps
-
Для дітей
-
Електроніка
-
Графіка / Відео / CAX
-
Ігри
-
Microsoft Office
-
Інструменти розробки
-
Програмування
-
Особистісний розвиток
-
Комп'ютерні мережі
-
Операційні системи
-
Тестування програмного забезпечення
-
Мобільні пристрої
-
UX/UI
-
Веброзробка, Web development
-
Управління
Подкасти
- Електронні книги
- Програмування
- Методи програмування
- OBJECT-ORIENTED PROGRAMMING
Деталі електронної книги
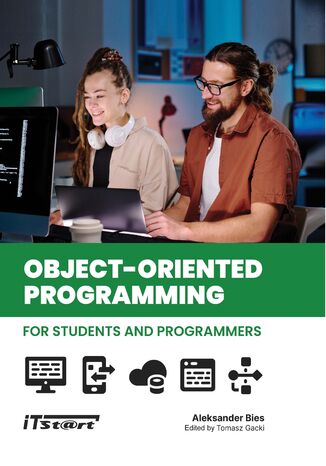
Object-Oriented Programming is a textbook that will enable any reader, regardless of their level, to understand the basic principles of structured and object-oriented programming. A beginner will have the opportunity to learn almost from scratch, and an advanced programmer will be able to consolidate, practice, and significantly expand his or her knowledge.
In the book, the author has gathered a large amount of basic programming concepts, explaining the concept of data, its types and more complex structures, i.e. collections, along with the ways of referring to them through names and pointers. He discussed conditional statements, iteration, functions, objects and classes, as well as inheritance, polymorphism and access rules. An additional advantage of the content discussed is the questions and exercises with answers and the source codes of all programs with the possibility of downloading them directly to the computer.
The author of the book is Aleksander Bies, a teacher of programming, a graduate of Computer Science and Applied Computer Science at the Faculty of Science and Technology of the University of Silesia, a doctoral candidate in the field of artificial intelligence methods in complex systems. He is the co-author of a machine learning model that won 2nd place in the international Orange Datahack with Energy competition.
Translations: Joanna Margowniczny an English teacher at Primary School No. 13 in Jaworzno and ZDZ in Sosnowiec, International IT ESSENTIALS instructor, OKE examiner.
TABLE OF CONTENTS
1 BEFORE YOU WRITE YOUR FIRST CODE... ................................................. 9
1.1 INTRODUCTION ...................................................................................................... 9
1.2 PROGRAMMING LANGUAGES AND THEIR DIVISION ......................................................... 9
1.2.1 Test yoursel! ............................................................................................ 13
1.3 DEVELOPMENT ENVIRONMENTS .............................................................................. 14
1.3.1 Rider ........................................................................................................ 15
1.3.2 Visual Studio ............................................................................................ 16
1.4 RIDER INSTALLATION ............................................................................................. 17
1.4.1 System Windows 11 ................................................................................ 17
1.4.2 System MacOS ......................................................................................... 21
1.4.3 System Ubuntu ........................................................................................ 23
1.4.4 Rider Initial configuration and first launch of the Rider environment ..... 25
1.5 MICROSOFT VISUAL STUDIO INSTALLATION ............................................................... 34
1.5.1 System Windows 11 ................................................................................ 34
1.5.2 Initial setup and first launch of Visual Studio .......................................... 37
1.6 C# LANGUAGE ..................................................................................................... 41
2 PROGRAMMING BASICS ........................................................................ 45
2.1 WE ARE WRITING THE FIRST PROGRAMME... .............................................................. 45
2.1.1 Test yourself! ........................................................................................... 47
2.1.2 Practical tasks: ........................................................................................ 49
2.2 BASIC OPERATIONS, COMMETS................................................................................ 49
2.2.1 Test yourself! ........................................................................................... 52
2.2.2 Practical tasks: ........................................................................................ 52
2.3 VARIABLES AND CONSTANTS, BASIC DATA TYPES, TYPE CONVERSION ............................... 53
2.3.1 Test yourself! ........................................................................................... 61
2.3.2 Practical tasks: ........................................................................................ 62
2.4 CONDITIONAL STATEMENTS ................................................................................... 63
2.4.1 If statement ............................................................................................. 66
2.4.2 The statement if … else ........................................................................... 68
2.4.3 Statement if … else if … else .................................................. 70
2.4.4 switch Statement ............................................................................... 73
2.4.5 Test yourself! ........................................................................................... 75
2.4.6 Pactical tasks: .......................................................................................... 76
2.5 LOOPS................................................................................................................ 76
2.5.1 For loop ................................................................................................... 77
2.5.2 A While loop ............................................................................................ 80
2.5.3 Do … while loop ....................................................................................... 85
2.5.4 Break and Continue commands .............................................................. 87
2.5.5 Nested loops ............................................................................................ 92
2.5.6 Test yourself! ........................................................................................... 95
2.5.7 Practical tasks: ........................................................................................ 96
2.6 RANGE OF VARIABLES ............................................................................................ 97
2.6.1 Test yourself! ......................................................................................... 103
2.7 COLLECTIONS .................................................................................................... 103
2.7.1 Arrays (one - dimensional) .................................................................... 104
2.7.2 Multidimensional arrays ....................................................................... 107
2.7.3 Irregular arrays ...................................................................................... 108
2.7.4 Lists ........................................................................................................ 110
2.7.5 Array List ............................................................................................... 113
2.7.6 Dictionaries ........................................................................................... 115
2.7.7 The "for" loop and collections ............................................................... 117
2.7.8 Foreach Loop ......................................................................................... 120
2.7.9 Test yourself! ......................................................................................... 124
2.7.10 Practical tasks: ...................................................................................... 125
3 „CLEAN CODE” ..................................................................................... 129
3.1.1 Test yourself! ......................................................................................... 136
4 FUNCTIONAL PROGRAMMING ............................................................. 141
4.1 WHAT IS A FUNCTION? ........................................................................................ 141
4.2 FURTHER ABOUT THE FUNCTIONS... ....................................................................... 144
4.2.1 Functions that return nothing ............................................................... 145
4.2.2 Functions that return numeric values .................................................... 146
4.2.3 Other types of functions ........................................................................ 150
4.2.4 Test yourself! ......................................................................................... 153
4.2.5 Practical tasks: ...................................................................................... 154
4.3 RECURSION ....................................................................................................... 155
4.3.1 What is recursion? ................................................................................. 155
4.3.2 Pros and cons of recursion..................................................................... 160
4.3.3 Test yourself! ......................................................................................... 161
4.3.4 Practical tasks: ...................................................................................... 162
5 "SYSTEM" NAMESPACE FUNCTIONS SYSTEM.NUMERIC LIBRARY ......... 165
5.1 BUILT-IN AND LIBRARY FUNCTION .......................................................................... 165
5.2 STRING CLASS FUNCTIONS .................................................................................... 166
5.3 FUNCTIONS THAT ALLOW YOU TO OPERATE ON COLLECTIONS ...................................... 177
5.4 MATH FUNCTIONS – MATH CLASS ........................................................................ 192
5.5 ELEMENT OF RANDOMNESS – FUNCTIONS OF THE RANDOM CLASS ............................... 195
5.6 SYSTEM.NUMERICS LIBRARY ................................................................................. 199
5.6.1 Big Integer ............................................................................................. 199
5.6.1 Vector2 .................................................................................................. 202
5.6.2 Vector3 .................................................................................................. 203
5.6.3 Test yourself! ......................................................................................... 205
6 GARBAGE COLLECTOR, POINTERS, STRUCTURES .................................. 209
6.1 GARBAGE COLLECTOR ......................................................................................... 209
6.2 POINTERS ......................................................................................................... 210
6.3 STRUCTURES ..................................................................................................... 214
6.3.1 Test yourself! ......................................................................................... 219
6.3.2 Practical tasks: ...................................................................................... 220
7 OBJECT-ORIENTED PROGRAMMING .................................................... 223
7.1 WHAT IS OBJECT-ORIENTED PROGRAMMING AND WHY IS IT WORTH PROGRAMMING THIS
WAY? 223
7.2 CLASS DEFINITION .............................................................................................. 224
7.3 CONSTRUCTOR AND DESTRUCTOR, CREATING OBJECTS ............................................... 226
7.3.1 Constructor ............................................................................................ 227
7.3.2 Destructor .............................................................................................. 228
7.3.3 Creating Objects .................................................................................... 229
7.4 METHODS......................................................................................................... 230
7.4.1 Calling methods on instances of a class ................................................ 232
7.4.2 Method overloading .............................................................................. 235
7.5 INHERITANCE AND POLYMORPHISM ........................................................................ 237
7.5.1 Inheritance mechanism ......................................................................... 237
7.5.2 Polymorphism ........................................................................................ 239
7.5.3 ToString Method ................................................................................... 246
7.6 ABSTRACT CLASSES AND INTERFACES ...................................................................... 249
7.6.1 What are abstract classes? ................................................................... 249
7.6.2 Interfaces ............................................................................................... 251
7.7 ACCESS MODIFIERS ............................................................................................. 252
7.7.1 Public ..................................................................................................... 253
7.7.2 Private ................................................................................................... 254
7.7.3 Protected ............................................................................................... 254
7.7.4 Internal .................................................................................................. 256
7.8 GET AND SET PROPERTIES AND ACCESSORIES ............................................................ 258
7.8.1 What are properties? Correct class design. ........................................... 258
Encapsulation. ....................................................................................................... 258
7.8.2 How do I use properties? ....................................................................... 260
7.8.3 Test yourself! ......................................................................................... 262
7.8.4 Practical tasks: ...................................................................................... 264
8 DELEGATES AND ANONYMOUS FUNCTIONS ........................................ 269
8.1 A FEW WORDS ABOUT THE DELEGATES ... ................................................................ 269
8.1.1 What are delegates and why are they used? Callback functions. ......... 269
8.1.2 Create delegates ................................................................................... 270
8.1.3 Delegates as callback functions. Generic delegates. ................ 271
8.1.4 Built-in delegates – Func and Action ..................................................... 275
8.2 ANONYMOUS FUNCTIONS AND LAMBDA EXPRESSIONS ............................................... 277
8.2.1 Test yourself! ......................................................................................... 280
8.2.2 Practical tasks: ...................................................................................... 281
9 EXCEPTIONS AND THEIR HANDLING ..................................................... 285
9.1 EXCEPTIONS ...................................................................................................... 285
9.2 EXCEPTION HANDLING –TRY, CATCH, FINALLY BLOCK ........................................ 289
9.2.1 Test yourself! ......................................................................................... 293
9.2.2 Practical tasks: ...................................................................................... 293
10 KEY ANSWERS ..................................................................................... 297
11 BIBLIOGRAPHY .................................................................................... 327
- Назва: OBJECT-ORIENTED PROGRAMMING
- Автор: Aleksander Bies
- Переклад: Joanna Margowniczy
- ISBN: 9788367989312, 9788367989312
- Дата видання: 2025-05-10
- Формат: Eлектронна книга
- Ідентифікатор видання: e_4gah
- Видавець: ITStart