Kategorie
Ebooki
-
Biznes i ekonomia
- Bitcoin
- Bizneswoman
- Coaching
- Controlling
- E-biznes
- Ekonomia
- Finanse
- Giełda i inwestycje
- Kompetencje osobiste
- Komputer w biurze
- Komunikacja i negocjacje
- Mała firma
- Marketing
- Motywacja
- Multimedialne szkolenia
- Nieruchomości
- Perswazja i NLP
- Podatki
- Polityka społeczna
- Poradniki
- Prezentacje
- Przywództwo
- Public Relation
- Raporty, analizy
- Sekret
- Social Media
- Sprzedaż
- Start-up
- Twoja kariera
- Zarządzanie
- Zarządzanie projektami
- Zasoby ludzkie (HR)
-
Dla dzieci
-
Dla młodzieży
-
Edukacja
-
Encyklopedie, słowniki
-
E-prasa
- Architektura i wnętrza
- BHP
- Biznes i Ekonomia
- Dom i ogród
- E-Biznes
- Finanse
- Finanse osobiste
- Firma
- Fotografia
- Informatyka
- Kadry i płace
- Komputery, Excel
- Księgowość
- Kultura i literatura
- Naukowe i akademickie
- Ochrona środowiska
- Opiniotwórcze
- Oświata
- Podatki
- Podróże
- Psychologia
- Religia
- Rolnictwo
- Rynek książki i prasy
- Transport i Spedycja
- Zdrowie i uroda
-
Historia
-
Informatyka
- Aplikacje biurowe
- Bazy danych
- Bioinformatyka
- Biznes IT
- CAD/CAM
- Digital Lifestyle
- DTP
- Elektronika
- Fotografia cyfrowa
- Grafika komputerowa
- Gry
- Hacking
- Hardware
- IT w ekonomii
- Pakiety naukowe
- Podręczniki szkolne
- Podstawy komputera
- Programowanie
- Programowanie mobilne
- Serwery internetowe
- Sieci komputerowe
- Start-up
- Systemy operacyjne
- Sztuczna inteligencja
- Technologia dla dzieci
- Webmasterstwo
-
Inne
-
Języki obce
-
Kultura i sztuka
-
Lektury szkolne
-
Literatura
- Antologie
- Ballada
- Biografie i autobiografie
- Dla dorosłych
- Dramat
- Dzienniki, pamiętniki, listy
- Epos, epopeja
- Esej
- Fantastyka i science-fiction
- Felietony
- Fikcja
- Humor, satyra
- Inne
- Klasyczna
- Kryminał
- Literatura faktu
- Literatura piękna
- Mity i legendy
- Nobliści
- Nowele
- Obyczajowa
- Okultyzm i magia
- Opowiadania
- Pamiętniki
- Podróże
- Poemat
- Poezja
- Polityka
- Popularnonaukowa
- Powieść
- Powieść historyczna
- Proza
- Przygodowa
- Publicystyka
- Reportaż
- Romans i literatura obyczajowa
- Sensacja
- Thriller, Horror
- Wywiady i wspomnienia
-
Nauki przyrodnicze
-
Nauki społeczne
-
Podręczniki szkolne
-
Popularnonaukowe i akademickie
- Archeologia
- Bibliotekoznawstwo
- Filmoznawstwo
- Filologia
- Filologia polska
- Filozofia
- Finanse i bankowość
- Geografia
- Gospodarka
- Handel. Gospodarka światowa
- Historia i archeologia
- Historia sztuki i architektury
- Kulturoznawstwo
- Lingwistyka
- Literaturoznawstwo
- Logistyka
- Matematyka
- Medycyna
- Nauki humanistyczne
- Pedagogika
- Pomoce naukowe
- Popularnonaukowa
- Pozostałe
- Psychologia
- Socjologia
- Teatrologia
- Teologia
- Teorie i nauki ekonomiczne
- Transport i spedycja
- Wychowanie fizyczne
- Zarządzanie i marketing
-
Poradniki
-
Poradniki do gier
-
Poradniki zawodowe i specjalistyczne
-
Prawo
- BHP
- Historia
- Kodeks drogowy. Prawo jazdy
- Nauki prawne
- Ochrona zdrowia
- Ogólne, kompendium wiedzy
- Podręczniki akademickie
- Pozostałe
- Prawo budowlane i lokalowe
- Prawo cywilne
- Prawo finansowe
- Prawo gospodarcze
- Prawo gospodarcze i handlowe
- Prawo karne
- Prawo karne. Przestępstwa karne. Kryminologia
- Prawo międzynarodowe
- Prawo międzynarodowe i zagraniczne
- Prawo ochrony zdrowia
- Prawo oświatowe
- Prawo podatkowe
- Prawo pracy i ubezpieczeń społecznych
- Prawo publiczne, konstytucyjne i administracyjne
- Prawo rodzinne i opiekuńcze
- Prawo rolne
- Prawo socjalne, prawo pracy
- Prawo Unii Europejskiej
- Przemysł
- Rolne i ochrona środowiska
- Słowniki i encyklopedie
- Zamówienia publiczne
- Zarządzanie
-
Przewodniki i podróże
- Afryka
- Albumy
- Ameryka Południowa
- Ameryka Środkowa i Północna
- Australia, Nowa Zelandia, Oceania
- Austria
- Azja
- Bałkany
- Bliski Wschód
- Bułgaria
- Chiny
- Chorwacja
- Czechy
- Dania
- Egipt
- Estonia
- Europa
- Francja
- Góry
- Grecja
- Hiszpania
- Holandia
- Islandia
- Litwa
- Łotwa
- Mapy, Plany miast, Atlasy
- Miniprzewodniki
- Niemcy
- Norwegia
- Podróże aktywne
- Polska
- Portugalia
- Pozostałe
- Przewodniki po hotelach i restauracjach
- Rosja
- Rumunia
- Słowacja
- Słowenia
- Szwajcaria
- Szwecja
- Świat
- Turcja
- Ukraina
- Węgry
- Wielka Brytania
- Włochy
-
Psychologia
- Filozofie życiowe
- Kompetencje psychospołeczne
- Komunikacja międzyludzka
- Mindfulness
- Ogólne
- Perswazja i NLP
- Psychologia akademicka
- Psychologia duszy i umysłu
- Psychologia pracy
- Relacje i związki
- Rodzicielstwo i psychologia dziecka
- Rozwiązywanie problemów
- Rozwój intelektualny
- Sekret
- Seksualność
- Uwodzenie
- Wygląd i wizerunek
- Życiowe filozofie
-
Religia
-
Sport, fitness, diety
-
Technika i mechanika
Audiobooki
-
Biznes i ekonomia
- Bitcoin
- Bizneswoman
- Coaching
- Controlling
- E-biznes
- Ekonomia
- Finanse
- Giełda i inwestycje
- Kompetencje osobiste
- Komunikacja i negocjacje
- Mała firma
- Marketing
- Motywacja
- Nieruchomości
- Perswazja i NLP
- Podatki
- Poradniki
- Prezentacje
- Przywództwo
- Public Relation
- Sekret
- Social Media
- Sprzedaż
- Start-up
- Twoja kariera
- Zarządzanie
- Zarządzanie projektami
- Zasoby ludzkie (HR)
-
Dla dzieci
-
Dla młodzieży
-
Edukacja
-
Encyklopedie, słowniki
-
Historia
-
Informatyka
-
Inne
-
Języki obce
-
Kultura i sztuka
-
Lektury szkolne
-
Literatura
- Antologie
- Ballada
- Biografie i autobiografie
- Dla dorosłych
- Dramat
- Dzienniki, pamiętniki, listy
- Epos, epopeja
- Esej
- Fantastyka i science-fiction
- Felietony
- Fikcja
- Humor, satyra
- Inne
- Klasyczna
- Kryminał
- Literatura faktu
- Literatura piękna
- Mity i legendy
- Nobliści
- Nowele
- Obyczajowa
- Okultyzm i magia
- Opowiadania
- Pamiętniki
- Podróże
- Poezja
- Polityka
- Popularnonaukowa
- Powieść
- Powieść historyczna
- Proza
- Przygodowa
- Publicystyka
- Reportaż
- Romans i literatura obyczajowa
- Sensacja
- Thriller, Horror
- Wywiady i wspomnienia
-
Nauki przyrodnicze
-
Nauki społeczne
-
Popularnonaukowe i akademickie
-
Poradniki
-
Poradniki zawodowe i specjalistyczne
-
Prawo
-
Przewodniki i podróże
-
Psychologia
- Filozofie życiowe
- Komunikacja międzyludzka
- Mindfulness
- Ogólne
- Perswazja i NLP
- Psychologia akademicka
- Psychologia duszy i umysłu
- Psychologia pracy
- Relacje i związki
- Rodzicielstwo i psychologia dziecka
- Rozwiązywanie problemów
- Rozwój intelektualny
- Sekret
- Seksualność
- Uwodzenie
- Wygląd i wizerunek
- Życiowe filozofie
-
Religia
-
Sport, fitness, diety
-
Technika i mechanika
Kursy video
-
Bazy danych
-
Big Data
-
Biznes, ekonomia i marketing
-
Cyberbezpieczeństwo
-
Data Science
-
DevOps
-
Dla dzieci
-
Elektronika
-
Grafika/Wideo/CAX
-
Gry
-
Microsoft Office
-
Narzędzia programistyczne
-
Programowanie
-
Rozwój osobisty
-
Sieci komputerowe
-
Systemy operacyjne
-
Testowanie oprogramowania
-
Urządzenia mobilne
-
UX/UI
-
Web development
-
Zarządzanie
Podcasty
- Ebooki
- Programowanie mobilne
- Android
- Kickstart Modern Android Development with Jetpack and Kotlin. Enhance your applications by integrating Jetpack and applying modern app architectural concepts
Szczegóły ebooka
Zaloguj się, jeśli jesteś zainteresowany treścią pozycji.
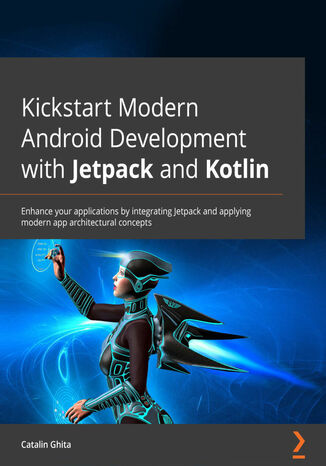
Kickstart Modern Android Development with Jetpack and Kotlin. Enhance your applications by integrating Jetpack and applying modern app architectural concepts
Ebook
With Jetpack libraries, you can build and design high-quality, robust Android apps that have an improved architecture and work consistently across different versions and devices. This book will help you understand how Jetpack allows developers to follow best practices and architectural patterns when building Android apps while also eliminating boilerplate code.
Developers working with Android and Kotlin will be able to put their knowledge to work with this condensed practical guide to building apps with the most popular Jetpack libraries, including Jetpack Compose, ViewModel, Hilt, Room, Paging, Lifecycle, and Navigation. You'll get to grips with relevant libraries and architectural patterns, including popular libraries in the Android ecosystem such as Retrofit, Coroutines, and Flow while building modern applications with real-world data.
By the end of this Android app development book, you'll have learned how to leverage Jetpack libraries and your knowledge of architectural concepts for building, designing, and testing robust Android applications for various use cases.
Developers working with Android and Kotlin will be able to put their knowledge to work with this condensed practical guide to building apps with the most popular Jetpack libraries, including Jetpack Compose, ViewModel, Hilt, Room, Paging, Lifecycle, and Navigation. You'll get to grips with relevant libraries and architectural patterns, including popular libraries in the Android ecosystem such as Retrofit, Coroutines, and Flow while building modern applications with real-world data.
By the end of this Android app development book, you'll have learned how to leverage Jetpack libraries and your knowledge of architectural concepts for building, designing, and testing robust Android applications for various use cases.
- 1. Creating Modern UIs with Jetpack Compose
- 2. Handling UI state with Jetpack ViewModel
- 3. Displaying Real Data from a REST API with Retrofit
- 4. Handling Async Operations with Coroutines
- 5. Adding Navigation in Compose with Jetpack Navigation
- 6. Adding Offline Capabilities with Jetpack Room
- 7. Introducing Presentation Patterns in Android
- 8. Getting Started with Clean Architecture in Android
- 9. Implementing Dependency Injection with Jetpack Hilt
- 10. Test your app with UI and Unit tests
- 11. Creating Infinite Lists with Jetpack Paging and Kotlin Flow
- 12. Exploring the Jetpack Lifecycle Components
- Tytuł: Kickstart Modern Android Development with Jetpack and Kotlin. Enhance your applications by integrating Jetpack and applying modern app architectural concepts
- Autor: Catalin Ghita
- Tytuł oryginału: Kickstart Modern Android Development with Jetpack and Kotlin. Enhance your applications by integrating Jetpack and applying modern app architectural concepts
- ISBN: 9781801818216, 9781801818216
- Data wydania: 2022-05-24
- Format: Ebook
- Identyfikator pozycji: e_2t3n
- Wydawca: Packt Publishing