Категорії
Електронні книги
-
Бізнес та економіка
- Біткойн
- Ділова жінка
- Коучинг
- Контроль
- Електронний бізнес
- Економіка
- Фінанси
- Фондова біржа та інвестиції
- Особисті компетенції
- Комп'ютер в офісі
- Комунікація та переговори
- Малий бізнес
- Маркетинг
- Мотивація
- Мультимедійне навчання
- Нерухомість
- Переконання та НЛП
- Податки
- Соціальна політика
- Порадники
- Презентації
- Лідерство
- Зв'язки з громадськістю
- Звіти, аналізи
- Секрет
- Соціальні засоби комунікації
- Продаж
- Стартап
- Ваша кар'єра
- Управління
- Управління проектами
- Людські ресурси (HR)
-
Для дітей
-
Для молоді
-
Освіта
-
Енциклопедії, словники
-
Електронна преса
- Architektura i wnętrza
- Biznes i Ekonomia
- Будинок та сад
- Електронний бізнес
- Фінанси
- Особисті фінанси
- Бізнес
- Фотографія
- Інформатика
- Відділ кадрів та оплата праці
- Комп'ютери, Excel
- Бухгалтерія
- Культура та література
- Наукові та академічні
- Охорона навколишнього середовища
- Впливові
- Освіта
- Податки
- Подорожі
- Психологія
- Релігія
- Сільське господарство
- Ринок книг і преси
- Транспорт та спедиція
- Здоров'я та краса
-
Історія
-
Інформатика
- Офісні застосунки
- Бази даних
- Біоінформатика
- Бізнес ІТ
- CAD/CAM
- Digital Lifestyle
- DTP
- Електроніка
- Цифрова фотографія
- Комп'ютерна графіка
- Ігри
- Хакування
- Hardware
- IT w ekonomii
- Наукові пакети
- Шкільні підручники
- Основи комп'ютера
- Програмування
- Мобільне програмування
- Інтернет-сервери
- Комп'ютерні мережі
- Стартап
- Операційні системи
- Штучний інтелект
- Технологія для дітей
- Вебмайстерність
-
Інше
-
Іноземні мови
-
Культура та мистецтво
-
Шкільні читанки
-
Література
- Антології
- Балада
- Біографії та автобіографії
- Для дорослих
- Драми
- Журнали, щоденники, листи
- Епос, епопея
- Нарис
- Наукова фантастика та фантастика
- Фельєтони
- Художня література
- Гумор, сатира
- Інше
- Класичний
- Кримінальний роман
- Нехудожня література
- Художня література
- Mity i legendy
- Лауреати Нобелівської премії
- Новели
- Побутовий роман
- Okultyzm i magia
- Оповідання
- Спогади
- Подорожі
- Оповідна поезія
- Поезія
- Політика
- Науково-популярна
- Роман
- Історичний роман
- Проза
- Пригодницька
- Журналістика
- Роман-репортаж
- Romans i literatura obyczajowa
- Сенсація
- Трилер, жах
- Інтерв'ю та спогади
-
Природничі науки
-
Соціальні науки
-
Шкільні підручники
-
Науково-популярна та академічна
- Археологія
- Bibliotekoznawstwo
- Кінознавство / Теорія кіно
- Філологія
- Польська філологія
- Філософія
- Finanse i bankowość
- Географія
- Економіка
- Торгівля. Світова економіка
- Історія та археологія
- Історія мистецтва і архітектури
- Культурологія
- Мовознавство
- літературні студії
- Логістика
- Математика
- Ліки
- Гуманітарні науки
- Педагогіка
- Навчальні засоби
- Науково-популярна
- Інше
- Психологія
- Соціологія
- Театральні студії
- Богослов’я
- Економічні теорії та науки
- Transport i spedycja
- Фізичне виховання
- Zarządzanie i marketing
-
Порадники
-
Ігрові посібники
-
Професійні та спеціальні порадники
-
Юридична
- Безпека життєдіяльності
- Історія
- Дорожній кодекс. Водійські права
- Юридичні науки
- Охорона здоров'я
- Загальне, компендіум
- Академічні підручники
- Інше
- Закон про будівництво і житло
- Цивільне право
- Фінансове право
- Господарське право
- Господарське та комерційне право
- Кримінальний закон
- Кримінальне право. Кримінальні злочини. Кримінологія
- Міжнародне право
- Міжнародне та іноземне право
- Закон про охорону здоров'я
- Закон про освіту
- Податкове право
- Трудове право та законодавство про соціальне забезпечення
- Громадське, конституційне та адміністративне право
- Кодекс про шлюб і сім'ю
- Аграрне право
- Соціальне право, трудове право
- Законодавство Євросоюзу
- Промисловість
- Сільське господарство та захист навколишнього середовища
- Словники та енциклопедії
- Державні закупівлі
- Управління
-
Путівники та подорожі
- Африка
- Альбоми
- Південна Америка
- Центральна та Північна Америка
- Австралія, Нова Зеландія, Океанія
- Австрія
- Азії
- Балкани
- Близький Схід
- Болгарія
- Китай
- Хорватія
- Чеська Республіка
- Данія
- Єгипет
- Естонія
- Європа
- Франція
- Гори
- Греція
- Іспанія
- Нідерланди
- Ісландія
- Литва
- Латвія
- Mapy, Plany miast, Atlasy
- Мініпутівники
- Німеччина
- Норвегія
- Активні подорожі
- Польща
- Португалія
- Інше
- Росія
- Румунія
- Словаччина
- Словенія
- Швейцарія
- Швеція
- Світ
- Туреччина
- Україна
- Угорщина
- Велика Британія
- Італія
-
Психологія
- Філософія життя
- Kompetencje psychospołeczne
- Міжособистісне спілкування
- Mindfulness
- Загальне
- Переконання та НЛП
- Академічна психологія
- Психологія душі та розуму
- Психологія праці
- Relacje i związki
- Батьківство та дитяча психологія
- Вирішення проблем
- Інтелектуальний розвиток
- Секрет
- Сексуальність
- Спокушання
- Зовнішній вигляд та імідж
- Філософія життя
-
Релігія
-
Спорт, фітнес, дієти
-
Техніка і механіка
Аудіокниги
-
Бізнес та економіка
- Біткойн
- Ділова жінка
- Коучинг
- Контроль
- Електронний бізнес
- Економіка
- Фінанси
- Фондова біржа та інвестиції
- Особисті компетенції
- Комунікація та переговори
- Малий бізнес
- Маркетинг
- Мотивація
- Нерухомість
- Переконання та НЛП
- Податки
- Порадники
- Презентації
- Лідерство
- Зв'язки з громадськістю
- Секрет
- Соціальні засоби комунікації
- Продаж
- Стартап
- Ваша кар'єра
- Управління
- Управління проектами
- Людські ресурси (HR)
-
Для дітей
-
Для молоді
-
Освіта
-
Енциклопедії, словники
-
Історія
-
Інформатика
-
Інше
-
Іноземні мови
-
Культура та мистецтво
-
Шкільні читанки
-
Література
- Антології
- Балада
- Біографії та автобіографії
- Для дорослих
- Драми
- Журнали, щоденники, листи
- Епос, епопея
- Нарис
- Наукова фантастика та фантастика
- Фельєтони
- Художня література
- Гумор, сатира
- Інше
- Класичний
- Кримінальний роман
- Нехудожня література
- Художня література
- Mity i legendy
- Лауреати Нобелівської премії
- Новели
- Побутовий роман
- Okultyzm i magia
- Оповідання
- Спогади
- Подорожі
- Поезія
- Політика
- Науково-популярна
- Роман
- Історичний роман
- Проза
- Пригодницька
- Журналістика
- Роман-репортаж
- Romans i literatura obyczajowa
- Сенсація
- Трилер, жах
- Інтерв'ю та спогади
-
Природничі науки
-
Соціальні науки
-
Науково-популярна та академічна
-
Порадники
-
Професійні та спеціальні порадники
-
Юридична
-
Путівники та подорожі
-
Психологія
- Філософія життя
- Міжособистісне спілкування
- Mindfulness
- Загальне
- Переконання та НЛП
- Академічна психологія
- Психологія душі та розуму
- Психологія праці
- Relacje i związki
- Батьківство та дитяча психологія
- Вирішення проблем
- Інтелектуальний розвиток
- Секрет
- Сексуальність
- Спокушання
- Зовнішній вигляд та імідж
- Філософія життя
-
Релігія
-
Спорт, фітнес, дієти
-
Техніка і механіка
Відеокурси
-
Бази даних
-
Big Data
-
Biznes, ekonomia i marketing
-
Кібербезпека
-
Data Science
-
DevOps
-
Для дітей
-
Електроніка
-
Графіка / Відео / CAX
-
Ігри
-
Microsoft Office
-
Інструменти розробки
-
Програмування
-
Особистісний розвиток
-
Комп'ютерні мережі
-
Операційні системи
-
Тестування програмного забезпечення
-
Мобільні пристрої
-
UX/UI
-
Веброзробка, Web development
-
Управління
Подкасти
- Електронні книги
- Інтернет-сервери
- Apache
- Building Big Data Pipelines with Apache Beam. Use a single programming model for both batch and stream data processing
Деталі електронної книги
Увійти, Якщо вас цікавить зміст видання.
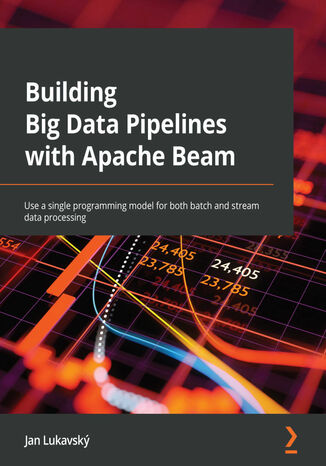
Building Big Data Pipelines with Apache Beam. Use a single programming model for both batch and stream data processing
Eлектронна книга
Apache Beam is an open source unified programming model for implementing and executing data processing pipelines, including Extract, Transform, and Load (ETL), batch, and stream processing.
This book will help you to confidently build data processing pipelines with Apache Beam. You’ll start with an overview of Apache Beam and understand how to use it to implement basic pipelines. You’ll also learn how to test and run the pipelines efficiently. As you progress, you’ll explore how to structure your code for reusability and also use various Domain Specific Languages (DSLs). Later chapters will show you how to use schemas and query your data using (streaming) SQL. Finally, you’ll understand advanced Apache Beam concepts, such as implementing your own I/O connectors.
By the end of this book, you’ll have gained a deep understanding of the Apache Beam model and be able to apply it to solve problems.
This book will help you to confidently build data processing pipelines with Apache Beam. You’ll start with an overview of Apache Beam and understand how to use it to implement basic pipelines. You’ll also learn how to test and run the pipelines efficiently. As you progress, you’ll explore how to structure your code for reusability and also use various Domain Specific Languages (DSLs). Later chapters will show you how to use schemas and query your data using (streaming) SQL. Finally, you’ll understand advanced Apache Beam concepts, such as implementing your own I/O connectors.
By the end of this book, you’ll have gained a deep understanding of the Apache Beam model and be able to apply it to solve problems.
- 1. Introduction to Data Processing with Apache Beam
- 2. Implementing, Testing, and Deploying Basic Pipelines
- 3. Implementing Pipelines Using Stateful Processing
- 4. Structuring Code for Reusability
- 5. Using SQL for Pipeline Implementation
- 6. Using Your Preferred Language with Portability
- 7. Extending Apache Beam's I/O Connectors
- 8. Understanding How Runners Execute Pipelines
- Назва: Building Big Data Pipelines with Apache Beam. Use a single programming model for both batch and stream data processing
- Автор: Jan Lukavský
- Оригінальна назва: Building Big Data Pipelines with Apache Beam. Use a single programming model for both batch and stream data processing
- ISBN: 9781800566569, 9781800566569
- Дата видання: 2022-01-21
- Формат: Eлектронна книга
- Ідентифікатор видання: e_2t49
- Видавець: Packt Publishing