Categories
Ebooks
-
Business and economy
- Bitcoin
- Businesswoman
- Coaching
- Controlling
- E-business
- Economy
- Finances
- Stocks and investments
- Personal competence
- Computer in the office
- Communication and negotiation
- Small company
- Marketing
- Motivation
- Multimedia trainings
- Real estate
- Persuasion and NLP
- Taxes
- Social policy
- Guides
- Presentations
- Leadership
- Public Relation
- Reports, analyses
- Secret
- Social Media
- Sales
- Start-up
- Your career
- Management
- Project management
- Human Resources
-
For children
-
For youth
-
Education
-
Encyclopedias, dictionaries
-
E-press
- Architektura i wnętrza
- Health and Safety
- Biznes i Ekonomia
- Home and garden
- E-business
- Ekonomia i finanse
- Finances
- Personal finance
- Business
- Photography
- Computer science
- HR & Payroll
- For women
- Computers, Excel
- Accounts
- Culture and literature
- Scientific and academic
- Environmental protection
- Opinion-forming
- Education
- Taxes
- Travelling
- Psychology
- Religion
- Agriculture
- Book and press market
- Transport and Spedition
- Healthand beauty
-
History
-
Computer science
- Office applications
- Data bases
- Bioinformatics
- IT business
- CAD/CAM
- Digital Lifestyle
- DTP
- Electronics
- Digital photography
- Computer graphics
- Games
- Hacking
- Hardware
- IT w ekonomii
- Scientific software package
- School textbooks
- Computer basics
- Programming
- Mobile programming
- Internet servers
- Computer networks
- Start-up
- Operational systems
- Artificial intelligence
- Technology for children
- Webmastering
-
Other
-
Foreign languages
-
Culture and art
-
School reading books
-
Literature
- Antology
- Ballade
- Biographies and autobiographies
- For adults
- Dramas
- Diaries, memoirs, letters
- Epic, epopee
- Essay
- Fantasy and science fiction
- Feuilletons
- Work of fiction
- Humour and satire
- Other
- Classical
- Crime fiction
- Non-fiction
- Fiction
- Mity i legendy
- Nobelists
- Novellas
- Moral
- Okultyzm i magia
- Short stories
- Memoirs
- Travelling
- Narrative poetry
- Poetry
- Politics
- Popular science
- Novel
- Historical novel
- Prose
- Adventure
- Journalism, publicism
- Reportage novels
- Romans i literatura obyczajowa
- Sensational
- Thriller, Horror
- Interviews and memoirs
-
Natural sciences
-
Social sciences
-
School textbooks
-
Popular science and academic
- Archeology
- Bibliotekoznawstwo
- Cinema studies
- Philology
- Polish philology
- Philosophy
- Finanse i bankowość
- Geography
- Economy
- Trade. World economy
- History and archeology
- History of art and architecture
- Cultural studies
- Linguistics
- Literary studies
- Logistics
- Maths
- Medicine
- Humanities
- Pedagogy
- Educational aids
- Popular science
- Other
- Psychology
- Sociology
- Theatre studies
- Theology
- Economic theories and teachings
- Transport i spedycja
- Physical education
- Zarządzanie i marketing
-
Guides
-
Game guides
-
Professional and specialist guides
-
Law
- Health and Safety
- History
- Road Code. Driving license
- Law studies
- Healthcare
- General. Compendium of knowledge
- Academic textbooks
- Other
- Construction and local law
- Civil law
- Financial law
- Economic law
- Economic and trade law
- Criminal law
- Criminal law. Criminal offenses. Criminology
- International law
- International law
- Health care law
- Educational law
- Tax law
- Labor and social security law
- Public, constitutional and administrative law
- Family and Guardianship Code
- agricultural law
- Social law, labour law
- European Union law
- Industry
- Agricultural and environmental
- Dictionaries and encyclopedia
- Public procurement
- Management
-
Tourist guides and travel
- Africa
- Albums
- Southern America
- North and Central America
- Australia, New Zealand, Oceania
- Austria
- Asia
- Balkans
- Middle East
- Bulgary
- China
- Croatia
- The Czech Republic
- Denmark
- Egipt
- Estonia
- Europe
- France
- Mountains
- Greece
- Spain
- Holand
- Iceland
- Lithuania
- Latvia
- Mapy, Plany miast, Atlasy
- Mini travel guides
- Germany
- Norway
- Active travelling
- Poland
- Portugal
- Other
- Przewodniki po hotelach i restauracjach
- Russia
- Romania
- Slovakia
- Slovenia
- Switzerland
- Sweden
- World
- Turkey
- Ukraine
- Hungary
- Great Britain
- Italy
-
Psychology
- Philosophy of life
- Kompetencje psychospołeczne
- Interpersonal communication
- Mindfulness
- General
- Persuasion and NLP
- Academic psychology
- Psychology of soul and mind
- Work psychology
- Relacje i związki
- Parenting and children psychology
- Problem solving
- Intellectual growth
- Secret
- Sexapeal
- Seduction
- Appearance and image
- Philosophy of life
-
Religion
-
Sport, fitness, diets
-
Technology and mechanics
Audiobooks
-
Business and economy
- Bitcoin
- Businesswoman
- Coaching
- Controlling
- E-business
- Economy
- Finances
- Stocks and investments
- Personal competence
- Communication and negotiation
- Small company
- Marketing
- Motivation
- Real estate
- Persuasion and NLP
- Taxes
- Social policy
- Guides
- Presentations
- Leadership
- Public Relation
- Secret
- Social Media
- Sales
- Start-up
- Your career
- Management
- Project management
- Human Resources
-
For children
-
For youth
-
Education
-
Encyclopedias, dictionaries
-
E-press
-
History
-
Computer science
-
Other
-
Foreign languages
-
Culture and art
-
School reading books
-
Literature
- Antology
- Ballade
- Biographies and autobiographies
- For adults
- Dramas
- Diaries, memoirs, letters
- Epic, epopee
- Essay
- Fantasy and science fiction
- Feuilletons
- Work of fiction
- Humour and satire
- Other
- Classical
- Crime fiction
- Non-fiction
- Fiction
- Mity i legendy
- Nobelists
- Novellas
- Moral
- Okultyzm i magia
- Short stories
- Memoirs
- Travelling
- Poetry
- Politics
- Popular science
- Novel
- Historical novel
- Prose
- Adventure
- Journalism, publicism
- Reportage novels
- Romans i literatura obyczajowa
- Sensational
- Thriller, Horror
- Interviews and memoirs
-
Natural sciences
-
Social sciences
-
Popular science and academic
-
Guides
-
Professional and specialist guides
-
Law
-
Tourist guides and travel
-
Psychology
- Philosophy of life
- Interpersonal communication
- Mindfulness
- General
- Persuasion and NLP
- Academic psychology
- Psychology of soul and mind
- Work psychology
- Relacje i związki
- Parenting and children psychology
- Problem solving
- Intellectual growth
- Secret
- Sexapeal
- Seduction
- Appearance and image
- Philosophy of life
-
Religion
-
Sport, fitness, diets
-
Technology and mechanics
Videocourses
-
Data bases
-
Big Data
-
Biznes, ekonomia i marketing
-
Cybersecurity
-
Data Science
-
DevOps
-
For children
-
Electronics
-
Graphics/Video/CAX
-
Games
-
Microsoft Office
-
Development tools
-
Programming
-
Personal growth
-
Computer networks
-
Operational systems
-
Software testing
-
Mobile devices
-
UX/UI
-
Web development
-
Management
Podcasts
- Ebooks
- Programming
- Java
- Kotlin Design Patterns and Best Practices. Build scalable applications using traditional, reactive, and concurrent design patterns in Kotlin - Second Edition
E-book details
Log in, If you're interested in the contents of the item.
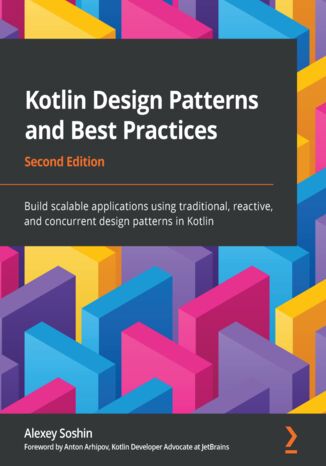
Kotlin Design Patterns and Best Practices. Build scalable applications using traditional, reactive, and concurrent design patterns in Kotlin - Second Edition
Ebook
This book shows you how easy it can be to implement traditional design patterns in the modern multi-paradigm Kotlin programming language, and takes you through the new patterns and paradigms that have emerged.
This second edition is updated to cover the changes introduced from Kotlin 1.2 up to 1.5 and focuses more on the idiomatic usage of coroutines, which have become a stable language feature. You'll begin by learning about the practical aspects of smarter coding in Kotlin, as well as understanding basic Kotlin syntax and the impact of design patterns on your code.
The book also provides an in-depth explanation of the classical design patterns, such as Creational, Structural, and Behavioral families, before moving on to functional programming. You'll go through reactive and concurrent patterns, and finally, get to grips with coroutines and structured concurrency to write performant, extensible, and maintainable code.
By the end of this Kotlin book, you'll have explored the latest trends in architecture and design patterns for microservices. You’ll also understand the tradeoffs when choosing between different architectures and make informed decisions.
This second edition is updated to cover the changes introduced from Kotlin 1.2 up to 1.5 and focuses more on the idiomatic usage of coroutines, which have become a stable language feature. You'll begin by learning about the practical aspects of smarter coding in Kotlin, as well as understanding basic Kotlin syntax and the impact of design patterns on your code.
The book also provides an in-depth explanation of the classical design patterns, such as Creational, Structural, and Behavioral families, before moving on to functional programming. You'll go through reactive and concurrent patterns, and finally, get to grips with coroutines and structured concurrency to write performant, extensible, and maintainable code.
By the end of this Kotlin book, you'll have explored the latest trends in architecture and design patterns for microservices. You’ll also understand the tradeoffs when choosing between different architectures and make informed decisions.
- 1. Getting Started with Kotlin
- 2. Working with Creational Patterns
- 3. Understanding Structural Patterns
- 4. Getting Familiar with Behavioral Patterns
- 5. Introducing Functional Programming
- 6. Threads and Coroutines
- 7. Controlling the Data Flow
- 8. Designing for Concurrency
- 9. Idioms and Anti-Patterns
- 10. Concurrent Microservices with Ktor
- 11. Reactive Microservices with Vert.x
- Title: Kotlin Design Patterns and Best Practices. Build scalable applications using traditional, reactive, and concurrent design patterns in Kotlin - Second Edition
- Author: Alexey Soshin, Anton Arhipov
- Original title: Kotlin Design Patterns and Best Practices. Build scalable applications using traditional, reactive, and concurrent design patterns in Kotlin - Second Edition
- ISBN: 9781801816281, 9781801816281
- Date of issue: 2022-01-21
- Format: Ebook
- Item ID: e_2t57
- Publisher: Packt Publishing