Categories
Ebooks
-
Business and economy
- Bitcoin
- Businesswoman
- Coaching
- Controlling
- E-business
- Economy
- Finances
- Stocks and investments
- Personal competence
- Computer in the office
- Communication and negotiation
- Small company
- Marketing
- Motivation
- Multimedia trainings
- Real estate
- Persuasion and NLP
- Taxes
- Social policy
- Guides
- Presentations
- Leadership
- Public Relation
- Reports, analyses
- Secret
- Social Media
- Sales
- Start-up
- Your career
- Management
- Project management
- Human Resources
-
For children
-
For youth
-
Education
-
Encyclopedias, dictionaries
-
E-press
- Architektura i wnętrza
- Health and Safety
- Biznes i Ekonomia
- Home and garden
- E-business
- Ekonomia i finanse
- Finances
- Personal finance
- Business
- Photography
- Computer science
- HR & Payroll
- For women
- Computers, Excel
- Accounts
- Culture and literature
- Scientific and academic
- Environmental protection
- Opinion-forming
- Education
- Taxes
- Travelling
- Psychology
- Religion
- Agriculture
- Book and press market
- Transport and Spedition
- Healthand beauty
-
History
-
Computer science
- Office applications
- Data bases
- Bioinformatics
- IT business
- CAD/CAM
- Digital Lifestyle
- DTP
- Electronics
- Digital photography
- Computer graphics
- Games
- Hacking
- Hardware
- IT w ekonomii
- Scientific software package
- School textbooks
- Computer basics
- Programming
- Mobile programming
- Internet servers
- Computer networks
- Start-up
- Operational systems
- Artificial intelligence
- Technology for children
- Webmastering
-
Other
-
Foreign languages
-
Culture and art
-
School reading books
-
Literature
- Antology
- Ballade
- Biographies and autobiographies
- For adults
- Dramas
- Diaries, memoirs, letters
- Epic, epopee
- Essay
- Fantasy and science fiction
- Feuilletons
- Work of fiction
- Humour and satire
- Other
- Classical
- Crime fiction
- Non-fiction
- Fiction
- Mity i legendy
- Nobelists
- Novellas
- Moral
- Okultyzm i magia
- Short stories
- Memoirs
- Travelling
- Narrative poetry
- Poetry
- Politics
- Popular science
- Novel
- Historical novel
- Prose
- Adventure
- Journalism, publicism
- Reportage novels
- Romans i literatura obyczajowa
- Sensational
- Thriller, Horror
- Interviews and memoirs
-
Natural sciences
-
Social sciences
-
School textbooks
-
Popular science and academic
- Archeology
- Bibliotekoznawstwo
- Cinema studies
- Philology
- Polish philology
- Philosophy
- Finanse i bankowość
- Geography
- Economy
- Trade. World economy
- History and archeology
- History of art and architecture
- Cultural studies
- Linguistics
- Literary studies
- Logistics
- Maths
- Medicine
- Humanities
- Pedagogy
- Educational aids
- Popular science
- Other
- Psychology
- Sociology
- Theatre studies
- Theology
- Economic theories and teachings
- Transport i spedycja
- Physical education
- Zarządzanie i marketing
-
Guides
-
Game guides
-
Professional and specialist guides
-
Law
- Health and Safety
- History
- Road Code. Driving license
- Law studies
- Healthcare
- General. Compendium of knowledge
- Academic textbooks
- Other
- Construction and local law
- Civil law
- Financial law
- Economic law
- Economic and trade law
- Criminal law
- Criminal law. Criminal offenses. Criminology
- International law
- International law
- Health care law
- Educational law
- Tax law
- Labor and social security law
- Public, constitutional and administrative law
- Family and Guardianship Code
- agricultural law
- Social law, labour law
- European Union law
- Industry
- Agricultural and environmental
- Dictionaries and encyclopedia
- Public procurement
- Management
-
Tourist guides and travel
- Africa
- Albums
- Southern America
- North and Central America
- Australia, New Zealand, Oceania
- Austria
- Asia
- Balkans
- Middle East
- Bulgary
- China
- Croatia
- The Czech Republic
- Denmark
- Egipt
- Estonia
- Europe
- France
- Mountains
- Greece
- Spain
- Holand
- Iceland
- Lithuania
- Latvia
- Mapy, Plany miast, Atlasy
- Mini travel guides
- Germany
- Norway
- Active travelling
- Poland
- Portugal
- Other
- Przewodniki po hotelach i restauracjach
- Russia
- Romania
- Slovakia
- Slovenia
- Switzerland
- Sweden
- World
- Turkey
- Ukraine
- Hungary
- Great Britain
- Italy
-
Psychology
- Philosophy of life
- Kompetencje psychospołeczne
- Interpersonal communication
- Mindfulness
- General
- Persuasion and NLP
- Academic psychology
- Psychology of soul and mind
- Work psychology
- Relacje i związki
- Parenting and children psychology
- Problem solving
- Intellectual growth
- Secret
- Sexapeal
- Seduction
- Appearance and image
- Philosophy of life
-
Religion
-
Sport, fitness, diets
-
Technology and mechanics
Audiobooks
-
Business and economy
- Bitcoin
- Businesswoman
- Coaching
- Controlling
- E-business
- Economy
- Finances
- Stocks and investments
- Personal competence
- Communication and negotiation
- Small company
- Marketing
- Motivation
- Real estate
- Persuasion and NLP
- Taxes
- Guides
- Presentations
- Leadership
- Public Relation
- Secret
- Social Media
- Sales
- Start-up
- Your career
- Management
- Project management
- Human Resources
-
For children
-
For youth
-
Education
-
Encyclopedias, dictionaries
-
E-press
-
History
-
Computer science
-
Other
-
Foreign languages
-
Culture and art
-
School reading books
-
Literature
- Antology
- Ballade
- Biographies and autobiographies
- For adults
- Dramas
- Diaries, memoirs, letters
- Epic, epopee
- Essay
- Fantasy and science fiction
- Feuilletons
- Work of fiction
- Humour and satire
- Other
- Classical
- Crime fiction
- Non-fiction
- Fiction
- Mity i legendy
- Nobelists
- Novellas
- Moral
- Okultyzm i magia
- Short stories
- Memoirs
- Travelling
- Poetry
- Politics
- Popular science
- Novel
- Historical novel
- Prose
- Adventure
- Journalism, publicism
- Reportage novels
- Romans i literatura obyczajowa
- Sensational
- Thriller, Horror
- Interviews and memoirs
-
Natural sciences
-
Social sciences
-
Popular science and academic
-
Guides
-
Professional and specialist guides
-
Law
-
Tourist guides and travel
-
Psychology
- Philosophy of life
- Interpersonal communication
- Mindfulness
- General
- Persuasion and NLP
- Academic psychology
- Psychology of soul and mind
- Work psychology
- Relacje i związki
- Parenting and children psychology
- Problem solving
- Intellectual growth
- Secret
- Sexapeal
- Seduction
- Appearance and image
- Philosophy of life
-
Religion
-
Sport, fitness, diets
-
Technology and mechanics
Videocourses
-
Data bases
-
Big Data
-
Biznes, ekonomia i marketing
-
Cybersecurity
-
Data Science
-
DevOps
-
For children
-
Electronics
-
Graphics/Video/CAX
-
Games
-
Microsoft Office
-
Development tools
-
Programming
-
Personal growth
-
Computer networks
-
Operational systems
-
Software testing
-
Mobile devices
-
UX/UI
-
Web development
-
Management
Podcasts
- Ebooks
- Programming
- C++
- Concurrency with Modern C++. What every professional C++ programmer should know about concurrency
E-book details
Log in, If you're interested in the contents of the item.
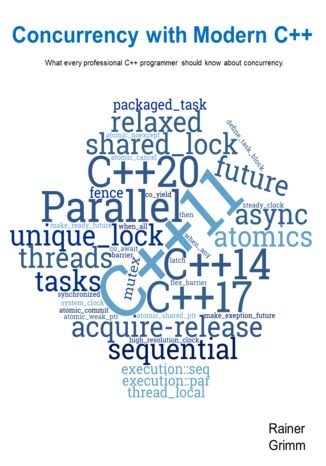
Concurrency with Modern C++. What every professional C++ programmer should know about concurrency
Ebook
C++11 is the first C++ standard that deals with concurrency. The story goes on with C++17 and will continue with C++20/23. Concurrency with Modern C++ is a practical guide that gets you to grips with concurrent programming in Modern C++.
Starting with the C++ memory model and using many ready-to-run code examples, the book covers everything you need to improve your C++ multithreading skills. You'll gain insight into different design patterns. You'll also uncover the general consideration you have to keep in mind while designing a concurrent data structure. The final chapter in the book talks extensively about the common pitfalls of concurrent programming and ways to overcome these hurdles.
By the end of the book, you'll have the skills to build your own concurrent programs and enhance your knowledge base.
Starting with the C++ memory model and using many ready-to-run code examples, the book covers everything you need to improve your C++ multithreading skills. You'll gain insight into different design patterns. You'll also uncover the general consideration you have to keep in mind while designing a concurrent data structure. The final chapter in the book talks extensively about the common pitfalls of concurrent programming and ways to overcome these hurdles.
By the end of the book, you'll have the skills to build your own concurrent programs and enhance your knowledge base.
- 1. A Quick Overview
- 2. The Details
- 3. Patterns
- 4. Data Structures
- 5. Further Information
- Title: Concurrency with Modern C++. What every professional C++ programmer should know about concurrency
- Author: Rainer Grimm
- Original title: Concurrency with Modern C++. What every professional C++ programmer should know about concurrency.
- ISBN: 9781838982737, 9781838982737
- Date of issue: 2019-12-30
- Format: Ebook
- Item ID: e_2t7p
- Publisher: Packt Publishing