Kategorie
Ebooki
-
Biznes i ekonomia
- Bitcoin
- Bizneswoman
- Coaching
- Controlling
- E-biznes
- Ekonomia
- Finanse
- Giełda i inwestycje
- Kompetencje osobiste
- Komputer w biurze
- Komunikacja i negocjacje
- Mała firma
- Marketing
- Motywacja
- Multimedialne szkolenia
- Nieruchomości
- Perswazja i NLP
- Podatki
- Polityka społeczna
- Poradniki
- Prezentacje
- Przywództwo
- Public Relation
- Raporty, analizy
- Sekret
- Social Media
- Sprzedaż
- Start-up
- Twoja kariera
- Zarządzanie
- Zarządzanie projektami
- Zasoby ludzkie (HR)
-
Dla dzieci
-
Dla młodzieży
-
Edukacja
-
Encyklopedie, słowniki
-
E-prasa
- Architektura i wnętrza
- Biznes i Ekonomia
- Dom i ogród
- E-Biznes
- Finanse
- Finanse osobiste
- Firma
- Fotografia
- Informatyka
- Kadry i płace
- Komputery, Excel
- Księgowość
- Kultura i literatura
- Naukowe i akademickie
- Ochrona środowiska
- Opiniotwórcze
- Oświata
- Podatki
- Podróże
- Psychologia
- Religia
- Rolnictwo
- Rynek książki i prasy
- Transport i Spedycja
- Zdrowie i uroda
-
Historia
-
Informatyka
- Aplikacje biurowe
- Bazy danych
- Bioinformatyka
- Biznes IT
- CAD/CAM
- Digital Lifestyle
- DTP
- Elektronika
- Fotografia cyfrowa
- Grafika komputerowa
- Gry
- Hacking
- Hardware
- IT w ekonomii
- Pakiety naukowe
- Podręczniki szkolne
- Podstawy komputera
- Programowanie
- Programowanie mobilne
- Serwery internetowe
- Sieci komputerowe
- Start-up
- Systemy operacyjne
- Sztuczna inteligencja
- Technologia dla dzieci
- Webmasterstwo
-
Inne
-
Języki obce
-
Kultura i sztuka
-
Lektury szkolne
-
Literatura
- Antologie
- Ballada
- Biografie i autobiografie
- Dla dorosłych
- Dramat
- Dzienniki, pamiętniki, listy
- Epos, epopeja
- Esej
- Fantastyka i science-fiction
- Felietony
- Fikcja
- Humor, satyra
- Inne
- Klasyczna
- Kryminał
- Literatura faktu
- Literatura piękna
- Mity i legendy
- Nobliści
- Nowele
- Obyczajowa
- Okultyzm i magia
- Opowiadania
- Pamiętniki
- Podróże
- Poemat
- Poezja
- Polityka
- Popularnonaukowa
- Powieść
- Powieść historyczna
- Proza
- Przygodowa
- Publicystyka
- Reportaż
- Romans i literatura obyczajowa
- Sensacja
- Thriller, Horror
- Wywiady i wspomnienia
-
Nauki przyrodnicze
-
Nauki społeczne
-
Podręczniki szkolne
-
Popularnonaukowe i akademickie
- Archeologia
- Bibliotekoznawstwo
- Filmoznawstwo
- Filologia
- Filologia polska
- Filozofia
- Finanse i bankowość
- Geografia
- Gospodarka
- Handel. Gospodarka światowa
- Historia i archeologia
- Historia sztuki i architektury
- Kulturoznawstwo
- Lingwistyka
- Literaturoznawstwo
- Logistyka
- Matematyka
- Medycyna
- Nauki humanistyczne
- Pedagogika
- Pomoce naukowe
- Popularnonaukowa
- Pozostałe
- Psychologia
- Socjologia
- Teatrologia
- Teologia
- Teorie i nauki ekonomiczne
- Transport i spedycja
- Wychowanie fizyczne
- Zarządzanie i marketing
-
Poradniki
-
Poradniki do gier
-
Poradniki zawodowe i specjalistyczne
-
Prawo
- BHP
- Historia
- Kodeks drogowy. Prawo jazdy
- Nauki prawne
- Ochrona zdrowia
- Ogólne, kompendium wiedzy
- Podręczniki akademickie
- Pozostałe
- Prawo budowlane i lokalowe
- Prawo cywilne
- Prawo finansowe
- Prawo gospodarcze
- Prawo gospodarcze i handlowe
- Prawo karne
- Prawo karne. Przestępstwa karne. Kryminologia
- Prawo międzynarodowe
- Prawo międzynarodowe i zagraniczne
- Prawo ochrony zdrowia
- Prawo oświatowe
- Prawo podatkowe
- Prawo pracy i ubezpieczeń społecznych
- Prawo publiczne, konstytucyjne i administracyjne
- Prawo rodzinne i opiekuńcze
- Prawo rolne
- Prawo socjalne, prawo pracy
- Prawo Unii Europejskiej
- Przemysł
- Rolne i ochrona środowiska
- Słowniki i encyklopedie
- Zamówienia publiczne
- Zarządzanie
-
Przewodniki i podróże
- Afryka
- Albumy
- Ameryka Południowa
- Ameryka Środkowa i Północna
- Australia, Nowa Zelandia, Oceania
- Austria
- Azja
- Bałkany
- Bliski Wschód
- Bułgaria
- Chiny
- Chorwacja
- Czechy
- Dania
- Egipt
- Estonia
- Europa
- Francja
- Góry
- Grecja
- Hiszpania
- Holandia
- Islandia
- Litwa
- Łotwa
- Mapy, Plany miast, Atlasy
- Miniprzewodniki
- Niemcy
- Norwegia
- Podróże aktywne
- Polska
- Portugalia
- Pozostałe
- Rosja
- Rumunia
- Słowacja
- Słowenia
- Szwajcaria
- Szwecja
- Świat
- Turcja
- Ukraina
- Węgry
- Wielka Brytania
- Włochy
-
Psychologia
- Filozofie życiowe
- Kompetencje psychospołeczne
- Komunikacja międzyludzka
- Mindfulness
- Ogólne
- Perswazja i NLP
- Psychologia akademicka
- Psychologia duszy i umysłu
- Psychologia pracy
- Relacje i związki
- Rodzicielstwo i psychologia dziecka
- Rozwiązywanie problemów
- Rozwój intelektualny
- Sekret
- Seksualność
- Uwodzenie
- Wygląd i wizerunek
- Życiowe filozofie
-
Religia
-
Sport, fitness, diety
-
Technika i mechanika
Audiobooki
-
Biznes i ekonomia
- Bitcoin
- Bizneswoman
- Coaching
- Controlling
- E-biznes
- Ekonomia
- Finanse
- Giełda i inwestycje
- Kompetencje osobiste
- Komunikacja i negocjacje
- Mała firma
- Marketing
- Motywacja
- Nieruchomości
- Perswazja i NLP
- Podatki
- Poradniki
- Prezentacje
- Przywództwo
- Public Relation
- Sekret
- Social Media
- Sprzedaż
- Start-up
- Twoja kariera
- Zarządzanie
- Zarządzanie projektami
- Zasoby ludzkie (HR)
-
Dla dzieci
-
Dla młodzieży
-
Edukacja
-
Encyklopedie, słowniki
-
Historia
-
Informatyka
-
Inne
-
Języki obce
-
Kultura i sztuka
-
Lektury szkolne
-
Literatura
- Antologie
- Ballada
- Biografie i autobiografie
- Dla dorosłych
- Dramat
- Dzienniki, pamiętniki, listy
- Epos, epopeja
- Esej
- Fantastyka i science-fiction
- Felietony
- Fikcja
- Humor, satyra
- Inne
- Klasyczna
- Kryminał
- Literatura faktu
- Literatura piękna
- Mity i legendy
- Nobliści
- Nowele
- Obyczajowa
- Okultyzm i magia
- Opowiadania
- Pamiętniki
- Podróże
- Poezja
- Polityka
- Popularnonaukowa
- Powieść
- Powieść historyczna
- Proza
- Przygodowa
- Publicystyka
- Reportaż
- Romans i literatura obyczajowa
- Sensacja
- Thriller, Horror
- Wywiady i wspomnienia
-
Nauki przyrodnicze
-
Nauki społeczne
-
Popularnonaukowe i akademickie
-
Poradniki
-
Poradniki zawodowe i specjalistyczne
-
Prawo
-
Przewodniki i podróże
-
Psychologia
- Filozofie życiowe
- Komunikacja międzyludzka
- Mindfulness
- Ogólne
- Perswazja i NLP
- Psychologia akademicka
- Psychologia duszy i umysłu
- Psychologia pracy
- Relacje i związki
- Rodzicielstwo i psychologia dziecka
- Rozwiązywanie problemów
- Rozwój intelektualny
- Sekret
- Seksualność
- Uwodzenie
- Wygląd i wizerunek
- Życiowe filozofie
-
Religia
-
Sport, fitness, diety
-
Technika i mechanika
Kursy video
-
Bazy danych
-
Big Data
-
Biznes, ekonomia i marketing
-
Cyberbezpieczeństwo
-
Data Science
-
DevOps
-
Dla dzieci
-
Elektronika
-
Grafika/Wideo/CAX
-
Gry
-
Microsoft Office
-
Narzędzia programistyczne
-
Programowanie
-
Rozwój osobisty
-
Sieci komputerowe
-
Systemy operacyjne
-
Testowanie oprogramowania
-
Urządzenia mobilne
-
UX/UI
-
Web development
-
Zarządzanie
Podcasty
- Ebooki
- Informatyka
- Programowanie
- Object-Oriented JavaScript
Szczegóły ebooka
Zaloguj się, jeśli jesteś zainteresowany treścią pozycji.
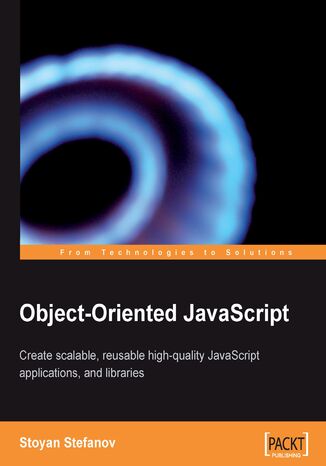
- Object-Oriented JavaScript
- Table of Contents
- Object-Oriented JavaScript
- Credits
- About the Author
- About the Reviewers
- Preface
- What This Book Covers
- Conventions
- Reader Feedback
- Customer Support
- Errata
- Questions
- 1. Introduction
- A Bit of History
- The Winds of Change
- The Present
- The Future
- Object-Oriented Programming
- Objects
- Classes
- Encapsulation
- Aggregation
- Inheritance
- Polymorphism
- OOP Summary
- Setting up Your Training Environment
- Getting the Tools You Need
- Using the Firebug Console
- Summary
- 2. Primitive Data Types, Arrays, Loops, and Conditions
- Variables
- Variables are Case Sensitive
- Operators
- Primitive Data Types
- Finding out the Value Type the typeof Operator
- Numbers
- Octal and Hexadecimal Numbers
- Exponent Literals
- Infinity
- NaN
- Strings
- String Conversions
- Special Strings
- Booleans
- Logical Operators
- Operator Precedence
- Lazy Evaluation
- Comparison
- Undefined and null
- Primitive Data Types Recap
- Arrays
- Adding/Updating Array Elements
- Deleting Elements
- Arrays of arrays
- Conditions and Loops
- Code Blocks
- if Conditions
- Checking if a Variable Exists
- Alternative if Syntax
- Switch
- Loops
- While Loops
- Do-while loops
- For Loops
- For-in Loops
- Comments
- Summary
- Exercises
- Variables
- 3. Functions
- What is a Function?
- Calling a Function
- Parameters
- Pre-defined Functions
- parseInt()
- parseFloat()
- isNaN()
- isFinite()
- Encode/Decode URIs
- eval()
- A Bonusthe alert() Function
- Scope of Variables
- Functions are Data
- Anonymous Functions
- Callback Functions
- Callback Examples
- Self-invoking Functions
- Inner (Private) Functions
- Functions that Return Functions
- Function, Rewrite Thyself!
- Closures
- Scope Chain
- Lexical Scope
- Breaking the Chain with a Closure
- Closure #1
- Closure #2
- A Definition and Closure #3
- Closures in a Loop
- Getter/Setter
- Iterator
- Summary
- Exercises
- What is a Function?
- 4. Objects
- From Arrays to Objects
- Elements, Properties, Methods
- Hashes, Associative Arrays
- Accessing Objects Properties
- Calling an Object's Methods
- Altering Properties/Methods
- Using this Value
- Constructor Functions
- The Global Object
- constructor Property
- instanceof Operator
- Functions that Return Objects
- Passing Objects
- Comparing Objects
- Objects in the Firebug Console
- Built-in Objects
- Object
- Array
- Interesting Array Methods
- Function
- Properties of the Function Objects
- Methods of the Function Objects
- The arguments Object Revisited
- Boolean
- Number
- String
- Interesting Methods of the String Objects
- Math
- Date
- Methods to Work with Date Objects
- RegExp
- Properties of the RegExp Objects
- Methods of the RegExp Objects
- String Methods that Accept Regular Expressions as Parameters
- search() and match()
- replace()
- Replace callbacks
- split()
- Passing a String When a regexp is Expected
- Error Objects
- Summary
- Exercises
- From Arrays to Objects
- 5. Prototype
- The prototype Property
- Adding Methods and Properties Using the Prototype
- Using the Prototype's Methods and Properties
- Own Properties versus prototype Properties
- Overwriting Prototype's Property with Own Property
- Enumerating Properties
- isPrototypeOf()
- The Secret __proto__ Link
- Augmenting Built-in Objects
- Augmenting Built-in ObjectsDiscussion
- Some Prototype gotchas
- Summary
- Exercises
- The prototype Property
- 6. Inheritance
- Prototype Chaining
- Prototype Chaining Example
- Moving Shared Properties to the Prototype
- Inheriting the Prototype Only
- A Temporary Constructornew F()
- UberAccess to the Parent from a Child Object
- Isolating the Inheritance Part into a Function
- Copying Properties
- Heads-up When Copying by Reference
- Objects Inherit from Objects
- Deep Copy
- object()
- Using a Mix of Prototypal Inheritance and Copying Properties
- Multiple Inheritance
- Mixins
- Parasitic Inheritance
- Borrowing a Constructor
- Borrow a Constructor and Copy its Prototype
- Summary
- Case Study: Drawing Shapes
- Analysis
- Implementation
- Testing
- Exercises
- Prototype Chaining
- 7. The Browser Environment
- Including JavaScript in an HTML Page
- BOM and DOMAn Overview
- BOM
- The window Object Revisited
- window.navigator
- Firebug as a Cheat Sheet
- window.location
- window.history
- window.frames
- window.screen
- window.open()/close()
- window.moveTo(), window.resizeTo()
- window.alert(), window.prompt(), window.confirm()
- window.setTimeout(), window.setInterval()
- window.document
- DOM
- Core DOM and HTML DOM
- Accessing DOM Nodes
- The document Node
- documentElement
- Child Nodes
- Attributes
- Accessing the Content Inside a Tag
- DOM Access Shortcuts
- Siblings, Body, First, and Last Child
- Walk the DOM
- Modifying DOM Nodes
- Modifying Styles
- Fun with Forms
- Creating New Nodes
- DOM-only Method
- cloneNode()
- insertBefore()
- Removing Nodes
- HTML-Only DOM Objects
- Primitive Ways to Access the Document
- document.write()
- Cookies, Title, Referrer, Domain
- Events
- Inline HTML Attributes
- Element Properties
- DOM Event Listeners
- Capturing and Bubbling
- Stop Propagation
- Prevent Default Behavior
- Cross-Browser Event Listeners
- Types of Events
- XMLHttpRequest
- Send the Request
- Process the Response
- Creating XMLHttpRequest Objects in IE prior to version 7
- A is for Asynchronous
- X is for XML
- An Example
- Summary
- Exercises
- 8. Coding and Design Patterns
- Coding Patterns
- Separating Behavior
- Content
- Presentation
- Behavior
- Example of Separating Behavior
- Namespaces
- An Object as a Namespace
- Namespaced Constructors
- A namespace() Method
- Init-Time Branching
- Lazy Definition
- Configuration Object
- Private Properties and Methods
- Privileged Methods
- Private Functions as Public Methods
- Self-Executing Functions
- Chaining
- JSON
- Separating Behavior
- Design Patterns
- Singleton
- Singleton 2
- Global Variable
- Property of the Constructor
- In a Private Property
- Factory
- Decorator
- Decorating a Christmas Tree
- Observer
- Summary
- Coding Patterns
- A. Reserved Words
- Keywords
- Future Reserved Words
- B. Built-in Functions
- C. Built-in Objects
- Object
- Members of the Object Constructor
- Members of the Objects Created by the Object Constructor
- Array
- Members of the Array Objects
- Function
- Members of the Function Objects
- Boolean
- Number
- Members of the Number Constructor
- Members of the Number Objects
- String
- Members of the String Constructor
- Members of the String Objects
- Date
- Members of the Date Constructor
- Members of the Date Objects
- Math
- Members of the Math Object
- RegExp
- Members of RegExp Objects
- Error Objects
- Members of the Error Objects
- Object
- D. Regular Expressions
- Index
- Tytuł: Object-Oriented JavaScript
- Autor: Stoyan Stefanov, Stoyan STEFANOV
- Tytuł oryginału: Object-Oriented JavaScript.
- ISBN: 9781847194152, 9781847194152
- Data wydania: 2008-07-23
- Format: Ebook
- Identyfikator pozycji: e_3ash
- Wydawca: Packt Publishing