Kategorie
Ebooki
-
Biznes i ekonomia
- Bitcoin
- Bizneswoman
- Coaching
- Controlling
- E-biznes
- Ekonomia
- Finanse
- Giełda i inwestycje
- Kompetencje osobiste
- Komputer w biurze
- Komunikacja i negocjacje
- Mała firma
- Marketing
- Motywacja
- Multimedialne szkolenia
- Nieruchomości
- Perswazja i NLP
- Podatki
- Polityka społeczna
- Poradniki
- Prezentacje
- Przywództwo
- Public Relation
- Raporty, analizy
- Sekret
- Social Media
- Sprzedaż
- Start-up
- Twoja kariera
- Zarządzanie
- Zarządzanie projektami
- Zasoby ludzkie (HR)
-
Dla dzieci
-
Dla młodzieży
-
Edukacja
-
Encyklopedie, słowniki
-
E-prasa
- Architektura i wnętrza
- Biznes i Ekonomia
- Dom i ogród
- E-Biznes
- Finanse
- Finanse osobiste
- Firma
- Fotografia
- Informatyka
- Kadry i płace
- Komputery, Excel
- Księgowość
- Kultura i literatura
- Naukowe i akademickie
- Ochrona środowiska
- Opiniotwórcze
- Oświata
- Podatki
- Podróże
- Psychologia
- Religia
- Rolnictwo
- Rynek książki i prasy
- Transport i Spedycja
- Zdrowie i uroda
-
Historia
-
Informatyka
- Aplikacje biurowe
- Bazy danych
- Bioinformatyka
- Biznes IT
- CAD/CAM
- Digital Lifestyle
- DTP
- Elektronika
- Fotografia cyfrowa
- Grafika komputerowa
- Gry
- Hacking
- Hardware
- IT w ekonomii
- Pakiety naukowe
- Podręczniki szkolne
- Podstawy komputera
- Programowanie
- Programowanie mobilne
- Serwery internetowe
- Sieci komputerowe
- Start-up
- Systemy operacyjne
- Sztuczna inteligencja
- Technologia dla dzieci
- Webmasterstwo
-
Inne
-
Języki obce
-
Kultura i sztuka
-
Lektury szkolne
-
Literatura
- Antologie
- Ballada
- Biografie i autobiografie
- Dla dorosłych
- Dramat
- Dzienniki, pamiętniki, listy
- Epos, epopeja
- Esej
- Fantastyka i science-fiction
- Felietony
- Fikcja
- Humor, satyra
- Inne
- Klasyczna
- Kryminał
- Literatura faktu
- Literatura piękna
- Mity i legendy
- Nobliści
- Nowele
- Obyczajowa
- Okultyzm i magia
- Opowiadania
- Pamiętniki
- Podróże
- Poemat
- Poezja
- Polityka
- Popularnonaukowa
- Powieść
- Powieść historyczna
- Proza
- Przygodowa
- Publicystyka
- Reportaż
- Romans i literatura obyczajowa
- Sensacja
- Thriller, Horror
- Wywiady i wspomnienia
-
Nauki przyrodnicze
-
Nauki społeczne
-
Podręczniki szkolne
-
Popularnonaukowe i akademickie
- Archeologia
- Bibliotekoznawstwo
- Filmoznawstwo
- Filologia
- Filologia polska
- Filozofia
- Finanse i bankowość
- Geografia
- Gospodarka
- Handel. Gospodarka światowa
- Historia i archeologia
- Historia sztuki i architektury
- Kulturoznawstwo
- Lingwistyka
- Literaturoznawstwo
- Logistyka
- Matematyka
- Medycyna
- Nauki humanistyczne
- Pedagogika
- Pomoce naukowe
- Popularnonaukowa
- Pozostałe
- Psychologia
- Socjologia
- Teatrologia
- Teologia
- Teorie i nauki ekonomiczne
- Transport i spedycja
- Wychowanie fizyczne
- Zarządzanie i marketing
-
Poradniki
-
Poradniki do gier
-
Poradniki zawodowe i specjalistyczne
-
Prawo
- BHP
- Historia
- Kodeks drogowy. Prawo jazdy
- Nauki prawne
- Ochrona zdrowia
- Ogólne, kompendium wiedzy
- Podręczniki akademickie
- Pozostałe
- Prawo budowlane i lokalowe
- Prawo cywilne
- Prawo finansowe
- Prawo gospodarcze
- Prawo gospodarcze i handlowe
- Prawo karne
- Prawo karne. Przestępstwa karne. Kryminologia
- Prawo międzynarodowe
- Prawo międzynarodowe i zagraniczne
- Prawo ochrony zdrowia
- Prawo oświatowe
- Prawo podatkowe
- Prawo pracy i ubezpieczeń społecznych
- Prawo publiczne, konstytucyjne i administracyjne
- Prawo rodzinne i opiekuńcze
- Prawo rolne
- Prawo socjalne, prawo pracy
- Prawo Unii Europejskiej
- Przemysł
- Rolne i ochrona środowiska
- Słowniki i encyklopedie
- Zamówienia publiczne
- Zarządzanie
-
Przewodniki i podróże
- Afryka
- Albumy
- Ameryka Południowa
- Ameryka Środkowa i Północna
- Australia, Nowa Zelandia, Oceania
- Austria
- Azja
- Bałkany
- Bliski Wschód
- Bułgaria
- Chiny
- Chorwacja
- Czechy
- Dania
- Egipt
- Europa
- Francja
- Góry
- Grecja
- Hiszpania
- Holandia
- Islandia
- Litwa
- Mapy, Plany miast, Atlasy
- Miniprzewodniki
- Niemcy
- Norwegia
- Podróże aktywne
- Polska
- Portugalia
- Pozostałe
- Rosja
- Rumunia
- Słowacja
- Słowenia
- Szwajcaria
- Szwecja
- Świat
- Turcja
- Ukraina
- Węgry
- Wielka Brytania
- Włochy
-
Psychologia
- Filozofie życiowe
- Kompetencje psychospołeczne
- Komunikacja międzyludzka
- Mindfulness
- Ogólne
- Perswazja i NLP
- Psychologia akademicka
- Psychologia duszy i umysłu
- Psychologia pracy
- Relacje i związki
- Rodzicielstwo i psychologia dziecka
- Rozwiązywanie problemów
- Rozwój intelektualny
- Sekret
- Seksualność
- Uwodzenie
- Wygląd i wizerunek
- Życiowe filozofie
-
Religia
-
Sport, fitness, diety
-
Technika i mechanika
Audiobooki
-
Biznes i ekonomia
- Bitcoin
- Bizneswoman
- Coaching
- Controlling
- E-biznes
- Ekonomia
- Finanse
- Giełda i inwestycje
- Kompetencje osobiste
- Komunikacja i negocjacje
- Mała firma
- Marketing
- Motywacja
- Nieruchomości
- Perswazja i NLP
- Podatki
- Poradniki
- Prezentacje
- Przywództwo
- Public Relation
- Sekret
- Social Media
- Sprzedaż
- Start-up
- Twoja kariera
- Zarządzanie
- Zarządzanie projektami
- Zasoby ludzkie (HR)
-
Dla dzieci
-
Dla młodzieży
-
Edukacja
-
Encyklopedie, słowniki
-
Historia
-
Informatyka
-
Inne
-
Języki obce
-
Kultura i sztuka
-
Lektury szkolne
-
Literatura
- Antologie
- Ballada
- Biografie i autobiografie
- Dla dorosłych
- Dramat
- Dzienniki, pamiętniki, listy
- Epos, epopeja
- Esej
- Fantastyka i science-fiction
- Felietony
- Fikcja
- Humor, satyra
- Inne
- Klasyczna
- Kryminał
- Literatura faktu
- Literatura piękna
- Mity i legendy
- Nobliści
- Nowele
- Obyczajowa
- Okultyzm i magia
- Opowiadania
- Pamiętniki
- Podróże
- Poezja
- Polityka
- Popularnonaukowa
- Powieść
- Powieść historyczna
- Proza
- Przygodowa
- Publicystyka
- Reportaż
- Romans i literatura obyczajowa
- Sensacja
- Thriller, Horror
- Wywiady i wspomnienia
-
Nauki przyrodnicze
-
Nauki społeczne
-
Popularnonaukowe i akademickie
-
Poradniki
-
Poradniki zawodowe i specjalistyczne
-
Prawo
-
Przewodniki i podróże
-
Psychologia
- Filozofie życiowe
- Komunikacja międzyludzka
- Mindfulness
- Ogólne
- Perswazja i NLP
- Psychologia akademicka
- Psychologia duszy i umysłu
- Psychologia pracy
- Relacje i związki
- Rodzicielstwo i psychologia dziecka
- Rozwiązywanie problemów
- Rozwój intelektualny
- Sekret
- Seksualność
- Uwodzenie
- Wygląd i wizerunek
- Życiowe filozofie
-
Religia
-
Sport, fitness, diety
-
Technika i mechanika
Kursy video
-
Bazy danych
-
Big Data
-
Biznes, ekonomia i marketing
-
Cyberbezpieczeństwo
-
Data Science
-
DevOps
-
Dla dzieci
-
Elektronika
-
Grafika/Wideo/CAX
-
Gry
-
Microsoft Office
-
Narzędzia programistyczne
-
Programowanie
-
Rozwój osobisty
-
Sieci komputerowe
-
Systemy operacyjne
-
Testowanie oprogramowania
-
Urządzenia mobilne
-
UX/UI
-
Web development
-
Zarządzanie
Podcasty
- Ebooki
- Programowanie
- Techniki programowania
- ASP.NET MVC 4 Mobile App Development. If your skill-sets include developing in C# on the .NET platform, this tutorial is a golden opportunity to extend your capabilities into mobile app development using the ASP.NET MVC framework. A totally practical primer
Szczegóły ebooka
Zaloguj się, jeśli jesteś zainteresowany treścią pozycji.
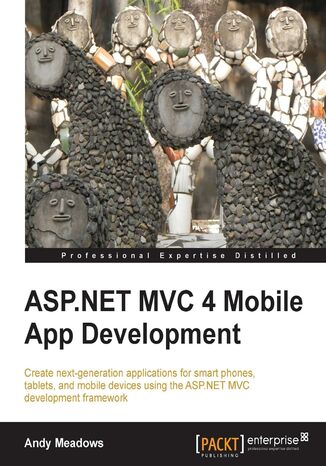
ASP.NET MVC 4 Mobile App Development. If your skill-sets include developing in C# on the .NET platform, this tutorial is a golden opportunity to extend your capabilities into mobile app development using the ASP.NET MVC framework. A totally practical primer
Andrew Scott Meadows, Andy Meadows
Ebook
The ASP.NET MVC 4 framework is used to build scalable web applications with the help of design patterns and .NET Framework. The Model-View-Controller (MVC) is a design principle which separates the components of a web application. This separation helps you to modify, develop, and test different components of a web application.
ASP.NET MVC 4 Mobile App Development helps you to develop next generation applications, while guiding you to deal with the constraints the mobile web places on application development. By the end of the book, you will be well versed with all the aspects of mobile app development.
ASP.NET MVC 4 Mobile App Development introduces you to developing mobile web apps using the ASP.NET MVC 4 framework. Walking you through the process of creating a homebrew recipe sharing application, this book teaches you the fundamentals and concepts relevant to developing Internet-ready mobile-enabled web apps. Through the sample application, you will learn how to secure your apps against XSS and CSRF attacks, open up your application to users using third party logins such as Google or Facebook, and how to use Razor, HTML 5, and CSS 3 to create custom views and content targeting mobile devices. Using these custom views, you will then learn how to create web apps with a native mobile device feel using jQuery mobile. By the end of the book, you will be presented with a set of challenges to prove to yourself that you now have the skills to extend your existing web applications to the mobile web or create new mobile web apps.
ASP.NET MVC 4 Mobile App Development helps you to develop next generation applications, while guiding you to deal with the constraints the mobile web places on application development. By the end of the book, you will be well versed with all the aspects of mobile app development.
ASP.NET MVC 4 Mobile App Development introduces you to developing mobile web apps using the ASP.NET MVC 4 framework. Walking you through the process of creating a homebrew recipe sharing application, this book teaches you the fundamentals and concepts relevant to developing Internet-ready mobile-enabled web apps. Through the sample application, you will learn how to secure your apps against XSS and CSRF attacks, open up your application to users using third party logins such as Google or Facebook, and how to use Razor, HTML 5, and CSS 3 to create custom views and content targeting mobile devices. Using these custom views, you will then learn how to create web apps with a native mobile device feel using jQuery mobile. By the end of the book, you will be presented with a set of challenges to prove to yourself that you now have the skills to extend your existing web applications to the mobile web or create new mobile web apps.
- ASP.NET MVC 4 Mobile App Development
- Table of Contents
- ASP.NET MVC 4 Mobile App Development
- Credits
- About the Author
- Acknowledgment
- About the Reviewers
- www.PacktPub.com
- Support files, eBooks, discount offers and more
- Why Subscribe?
- Free Access for Packt account holders
- Instant Updates on New Packt Books
- Preface
- What this book covers
- What you need for this book
- Who this book is for
- Conventions
- Reader feedback
- Customer support
- Downloading the example code
- Errata
- Piracy
- Questions
- 1. Developing for the Mobile Web
- History of the mobile web
- The Nokia 9000
- Market fragmentation
- WAP 1.0 and WML
- WAP 2.0 and XHTML MP
- Continued development constraints
- Processing constraints
- Network constraints
- Content compression
- Server to client compression
- Minification
- Image optimizations
- Lower color depth
- CSS image sprites
- Data URIs
- Content Delivery Networks
- Cached data
- Less traffic
- Content compression
- Presentation constraints
- Single window
- Lower resolution
- Content spacing
- Viewing the mobile web
- Market percentage
- Browser variants and compatibility
- WebKit
- Trident
- Gecko
- Presto
- Emulating the mobile web
- Mobile device and browser emulators
- Opera
- Android
- iOS
- Windows Mobile
- The user agent
- Emulating Internet Explorer Mobile
- Emulating Mobile Safari
- Emulating Chrome for Mobile
- Emulation in this book
- Mobile device and browser emulators
- Support for the mobile web in ASP.NET MVC 4
- Summary
- History of the mobile web
- 2. Homebrew and You
- Understanding the homebrew domain
- Knowing your ingredients
- Malt
- Yeast
- Ale versus lager
- Hops
- Brewing
- Mashing
- Sparging
- The boil
- Fermentation
- Bottling and kegging
- Knowing your ingredients
- About our mobile app
- App requirements
- Adding, editing, and deleting recipes
- Adding recipes to a library
- Rating recipes
- Commenting on recipes
- Anonymous browsing, authenticated contributing
- App requirements
- The BrewHow solution
- Creating the project
- Choosing our template
- The Empty template
- The Basic template
- The Internet Application template
- The Intranet Application template
- The Mobile Application template
- The Web API template
- Project changes in MVC 4
- NuGet
- Global.asax
- Launching the BrewHow app
- Responsive design
- Configuring and launching an emulator
- Summary
- Understanding the homebrew domain
- 3. Introducing ASP.NET MVC 4
- The Model-View-Controller pattern
- The controller
- The view
- The model
- The MVC pattern and ASP.NET MVC 4
- Controllers in ASP.NET MVC
- Creating the Recipe controller
- Introduction to routing
- Action methods
- ActionResults
- Invoking the Recipe controller
- Views in ASP.NET MVC
- Razor
- The @ character
- Code blocks
- Expressions
- Inline code
- Comments
- The @ character
- Shared views
- Layouts
- The _ViewStart file
- Partial views
- HTML helpers
- Html.RenderPartial and Html.Partial
- Html.RenderAction and Html.Action
- Display templates
- Html.Display
- Html.DisplayFor
- Html.DisplayForModel
- Editor templates
- Creating our Recipe view
- Making Recipe default
- Razor
- Returning a model to the view
- Using ViewData
- Using ViewBag
- Using TempData
- Strongly typed models
- Returning a Recipe list
- Creating the model
- Returning the model
- Displaying the model
- Controllers in ASP.NET MVC
- Summary
- The Model-View-Controller pattern
- 4. Modeling BrewHow in EF5
- Whats new in Entity Framework 5.0?
- Performance enhancements
- LocalDB support
- Enumeration support
- The BrewHow model
- Modeling data
- Recipe
- Review
- Style
- Category
- The BrewHow context
- Generating our database
- Altering the model
- Adding relationships
- Overriding conventions
- Enabling migrations
- The InitialCreate migration
- The Configuration class
- Adding seed data
- Adding a migration
- Applying migrations
- Consuming the model
- Pagination
- Modeling data
- Summary
- Whats new in Entity Framework 5.0?
- 5. The BrewHow Domain and Domain-driven Design
- Tenets of DDD
- Domain model
- Entities
- Value objects
- Aggregates
- Factories
- Repositories
- Services
- BrewHow design
- BrewHow entities
- BrewHow repositories
- Consuming the domain
- Recipe view model
- Data annotations
- Recipe controller
- GET versus POST
- Model binding
- Recipe views
- Recipe view model
- Summary
- Tenets of DDD
- 6. Writing Maintainable Code
- The SOLID principles
- Single Responsibility Principle
- Open Closed Principle
- Liskov Substitution Principle
- Interface Segregation Principle
- Dependency Inversion Principle
- SOLIDifying BrewHow
- Adding interfaces
- Infrastructure
- Dependency Injection
- Service locator
- Managed Extensibility Framework
- Convention-based configuration
- MEF Service Locator
- Using the MEF Service Locator
- Managed Extensibility Framework
- Dependency Resolver
- The MefDependencyResolver class
- Completing the conversion
- IBrewHowContext
- Repositories
- Registering dependencies
- Adding interfaces
- Summary
- The SOLID principles
- 7. Separating Functionality Using Routes and Areas
- Routes
- Locating by style
- Routing constraints
- Style interaction
- Recipe list modification
- Style Controller and view
- Slugging BrewHow
- Model slugs
- Stage the database
- Modifying entities
- Retrieval by slug
- Model slugs
- Locating by style
- Areas
- Creating the review area
- Registering the Review area
- The Recipe review controller
- Recipe review view models
- Recipe review action methods
- Creating the views
- Area route values
- Routing namespaces
- Summary
- Routes
- 8. Validating User Input
- Data validation
- Data annotations
- MetadataType attribute
- Updating the database
- Validating the validations
- Server validation
- Data annotations
- Cross-Site Request Forgery (CSRF)
- ValidateAntiForgeryToken
- Cross-Site Scripting (XSS)
- ValidateInput attribute
- AllowHtml
- Html.Raw
- Summary
- Data validation
- 9. Identifying and Authorizing Users
- User authentication
- Windows authentication
- Forms authentication
- Authenticating BrewHow users
- SimpleMembership
- Customizing authentication
- SimpleMembership initialization
- Unifying contexts
- The UserProfile repository
- AccountController contexts
- Registering and logging in
- External authentication
- Registering with an external account
- Associating an external account
- Authorization
- Restricting access
- The Authorize attribute
- Authorizing user contributions
- Cleaning the UI
- Content ownership
- Enabling ownership
- UserProfile schema mapping
- Seeding users
- Applying the ownership migration
- Assigning ownership
- Enforcing ownership
- Adjusting the view model
- Ensuring ownership
- Validating ownership
- Enabling ownership
- A recipe library
- The library data model
- The library repository
- The library controller
- The library view
- Restricting access
- Summary
- User authentication
- 10. Asynchronous Programming and Bundles
- Asynchronous programming
- Task Parallel Library
- Task
- Creating a Task
- Awaiting completion
- Completion callbacks
- Task
- Async
- Await
- Asynchronous controller action methods
- Creating asynchronous actions
- An asynchronous recipe controller
- Task Parallel Library
- Bundles
- Creating bundles
- Bundle types
- Wildcard support
- Consuming bundles
- Creating bundles
- Summary
- Asynchronous programming
- 11. Coding for the Real-time Web
- Simulating a connected state
- Long polling
- Forever Frame
- Server-Sent Events
- WebSockets
- SignalR
- Persistent connections
- Hubs
- Real-time recipe updates
- Installing and configuring SignalR
- Creating the recipe hub
- Modifying the recipe list view
- Publishing event notifications
- Summary
- Simulating a connected state
- 12. Designing Your App for Mobile Devices
- HTML5
- Markup changes
- The DOCTYPE tag
- The character set
- Type attributes
- Visual Studio 2012 support
- Semantic tags
- The article tag
- The header tag
- The section tag
- The nav tag
- The footer tag
- Modifying recipe details
- Custom data attributes
- Form controls
- Local storage
- Geolocation
- Markup changes
- CSS3
- Media types
- CSS selectors
- Type selectors
- ID selectors
- Attribute selectors
- Class selectors
- Universal selectors
- Pseudo-class selectors
- CSS media queries
- Media features
- The viewport meta tag
- A responsive design
- A responsive list
- Summary
- HTML5
- 13. Extending Support for the Mobile Web
- Mobile views
- A .Mobile layout
- Mobilizing BrewHow
- Removing content
- Prioritizing content
- How it works
- Display modes
- Supporting Asus Nexus 7
- Creating the display mode
- Registering the display mode
- Testing with Nexus 7
- Summary
- Mobile views
- 14. Improving the User Experience with jQuery Mobile
- Installing jQuery Mobile
- Enabling the jQuery Mobile bundle
- Viewing the results
- jQuery Mobile's layout
- Data-roles and data attributes
- Form elements
- Themes
- $.mobile
- View switcher
- Mobilizing BrewHow
- Adjusting the header
- The home button
- Logging in users
- Site navigation
- Creating a footer
- Desktop footer
- Configuring content
- Recipe list
- The jQuery Mobile listview
- Expanded listview content
- Listview filters
- Buttons
- Navigation hints
- Recipe details
- Back button
- Action buttons
- Recipe edits
- Fieldcontain
- Reviews
- IsMobileDevice
- Mobile views
- Recipe list
- Adjusting the header
- Summary
- Installing jQuery Mobile
- 15. Reader Challenges
- Full-text search
- Embedded search
- Search boxes
- APIs
- Lucene.NET
- SQL Server Full-text Search
- Embedded search
- Socialization
- Social media support
- Recipe additions
- Recipe sharing
- Offline support
- Push notifications
- Social media support
- Going native
- ASP.NET Web API
- Developing native apps
- PhoneGap and Appcelerator
- Xamarin
- Summary
- Full-text search
- Index
- Tytuł: ASP.NET MVC 4 Mobile App Development. If your skill-sets include developing in C# on the .NET platform, this tutorial is a golden opportunity to extend your capabilities into mobile app development using the ASP.NET MVC framework. A totally practical primer
- Autor: Andrew Scott Meadows, Andy Meadows
- Tytuł oryginału: ASP.NET MVC 4 Mobile App Development. If your skill-sets include developing in C# on the .NET platform, this tutorial is a golden opportunity to extend your capabilities into mobile app development using the ASP.NET MVC framework. A totally practical primer.
- ISBN: 9781849687379, 9781849687379
- Data wydania: 2013-07-23
- Format: Ebook
- Identyfikator pozycji: e_3cim
- Wydawca: Packt Publishing