Kategorie
Ebooki
-
Biznes i ekonomia
- Bitcoin
- Bizneswoman
- Coaching
- Controlling
- E-biznes
- Ekonomia
- Finanse
- Giełda i inwestycje
- Kompetencje osobiste
- Komputer w biurze
- Komunikacja i negocjacje
- Mała firma
- Marketing
- Motywacja
- Multimedialne szkolenia
- Nieruchomości
- Perswazja i NLP
- Podatki
- Polityka społeczna
- Poradniki
- Prezentacje
- Przywództwo
- Public Relation
- Raporty, analizy
- Sekret
- Social Media
- Sprzedaż
- Start-up
- Twoja kariera
- Zarządzanie
- Zarządzanie projektami
- Zasoby ludzkie (HR)
-
Dla dzieci
-
Dla młodzieży
-
Edukacja
-
Encyklopedie, słowniki
-
E-prasa
- Architektura i wnętrza
- Biznes i Ekonomia
- Dom i ogród
- E-Biznes
- Finanse
- Finanse osobiste
- Firma
- Fotografia
- Informatyka
- Kadry i płace
- Komputery, Excel
- Księgowość
- Kultura i literatura
- Naukowe i akademickie
- Ochrona środowiska
- Opiniotwórcze
- Oświata
- Podatki
- Podróże
- Psychologia
- Religia
- Rolnictwo
- Rynek książki i prasy
- Transport i Spedycja
- Zdrowie i uroda
-
Historia
-
Informatyka
- Aplikacje biurowe
- Bazy danych
- Bioinformatyka
- Biznes IT
- CAD/CAM
- Digital Lifestyle
- DTP
- Elektronika
- Fotografia cyfrowa
- Grafika komputerowa
- Gry
- Hacking
- Hardware
- IT w ekonomii
- Pakiety naukowe
- Podręczniki szkolne
- Podstawy komputera
- Programowanie
- Programowanie mobilne
- Serwery internetowe
- Sieci komputerowe
- Start-up
- Systemy operacyjne
- Sztuczna inteligencja
- Technologia dla dzieci
- Webmasterstwo
-
Inne
-
Języki obce
-
Kultura i sztuka
-
Lektury szkolne
-
Literatura
- Antologie
- Ballada
- Biografie i autobiografie
- Dla dorosłych
- Dramat
- Dzienniki, pamiętniki, listy
- Epos, epopeja
- Esej
- Fantastyka i science-fiction
- Felietony
- Fikcja
- Humor, satyra
- Inne
- Klasyczna
- Kryminał
- Literatura faktu
- Literatura piękna
- Mity i legendy
- Nobliści
- Nowele
- Obyczajowa
- Okultyzm i magia
- Opowiadania
- Pamiętniki
- Podróże
- Poemat
- Poezja
- Polityka
- Popularnonaukowa
- Powieść
- Powieść historyczna
- Proza
- Przygodowa
- Publicystyka
- Reportaż
- Romans i literatura obyczajowa
- Sensacja
- Thriller, Horror
- Wywiady i wspomnienia
-
Nauki przyrodnicze
-
Nauki społeczne
-
Podręczniki szkolne
-
Popularnonaukowe i akademickie
- Archeologia
- Bibliotekoznawstwo
- Filmoznawstwo
- Filologia
- Filologia polska
- Filozofia
- Finanse i bankowość
- Geografia
- Gospodarka
- Handel. Gospodarka światowa
- Historia i archeologia
- Historia sztuki i architektury
- Kulturoznawstwo
- Lingwistyka
- Literaturoznawstwo
- Logistyka
- Matematyka
- Medycyna
- Nauki humanistyczne
- Pedagogika
- Pomoce naukowe
- Popularnonaukowa
- Pozostałe
- Psychologia
- Socjologia
- Teatrologia
- Teologia
- Teorie i nauki ekonomiczne
- Transport i spedycja
- Wychowanie fizyczne
- Zarządzanie i marketing
-
Poradniki
-
Poradniki do gier
-
Poradniki zawodowe i specjalistyczne
-
Prawo
- BHP
- Historia
- Kodeks drogowy. Prawo jazdy
- Nauki prawne
- Ochrona zdrowia
- Ogólne, kompendium wiedzy
- Podręczniki akademickie
- Pozostałe
- Prawo budowlane i lokalowe
- Prawo cywilne
- Prawo finansowe
- Prawo gospodarcze
- Prawo gospodarcze i handlowe
- Prawo karne
- Prawo karne. Przestępstwa karne. Kryminologia
- Prawo międzynarodowe
- Prawo międzynarodowe i zagraniczne
- Prawo ochrony zdrowia
- Prawo oświatowe
- Prawo podatkowe
- Prawo pracy i ubezpieczeń społecznych
- Prawo publiczne, konstytucyjne i administracyjne
- Prawo rodzinne i opiekuńcze
- Prawo rolne
- Prawo socjalne, prawo pracy
- Prawo Unii Europejskiej
- Przemysł
- Rolne i ochrona środowiska
- Słowniki i encyklopedie
- Zamówienia publiczne
- Zarządzanie
-
Przewodniki i podróże
- Afryka
- Albumy
- Ameryka Południowa
- Ameryka Środkowa i Północna
- Australia, Nowa Zelandia, Oceania
- Austria
- Azja
- Bałkany
- Bliski Wschód
- Bułgaria
- Chiny
- Chorwacja
- Czechy
- Dania
- Egipt
- Europa
- Francja
- Góry
- Grecja
- Hiszpania
- Holandia
- Islandia
- Litwa
- Mapy, Plany miast, Atlasy
- Miniprzewodniki
- Niemcy
- Norwegia
- Podróże aktywne
- Polska
- Portugalia
- Pozostałe
- Rosja
- Rumunia
- Słowacja
- Słowenia
- Szwajcaria
- Szwecja
- Świat
- Turcja
- Ukraina
- Węgry
- Wielka Brytania
- Włochy
-
Psychologia
- Filozofie życiowe
- Kompetencje psychospołeczne
- Komunikacja międzyludzka
- Mindfulness
- Ogólne
- Perswazja i NLP
- Psychologia akademicka
- Psychologia duszy i umysłu
- Psychologia pracy
- Relacje i związki
- Rodzicielstwo i psychologia dziecka
- Rozwiązywanie problemów
- Rozwój intelektualny
- Sekret
- Seksualność
- Uwodzenie
- Wygląd i wizerunek
- Życiowe filozofie
-
Religia
-
Sport, fitness, diety
-
Technika i mechanika
Audiobooki
-
Biznes i ekonomia
- Bitcoin
- Bizneswoman
- Coaching
- Controlling
- E-biznes
- Ekonomia
- Finanse
- Giełda i inwestycje
- Kompetencje osobiste
- Komunikacja i negocjacje
- Mała firma
- Marketing
- Motywacja
- Nieruchomości
- Perswazja i NLP
- Podatki
- Poradniki
- Prezentacje
- Przywództwo
- Public Relation
- Sekret
- Social Media
- Sprzedaż
- Start-up
- Twoja kariera
- Zarządzanie
- Zarządzanie projektami
- Zasoby ludzkie (HR)
-
Dla dzieci
-
Dla młodzieży
-
Edukacja
-
Encyklopedie, słowniki
-
Historia
-
Informatyka
-
Inne
-
Języki obce
-
Kultura i sztuka
-
Lektury szkolne
-
Literatura
- Antologie
- Ballada
- Biografie i autobiografie
- Dla dorosłych
- Dramat
- Dzienniki, pamiętniki, listy
- Epos, epopeja
- Esej
- Fantastyka i science-fiction
- Felietony
- Fikcja
- Humor, satyra
- Inne
- Klasyczna
- Kryminał
- Literatura faktu
- Literatura piękna
- Mity i legendy
- Nobliści
- Nowele
- Obyczajowa
- Okultyzm i magia
- Opowiadania
- Pamiętniki
- Podróże
- Poezja
- Polityka
- Popularnonaukowa
- Powieść
- Powieść historyczna
- Proza
- Przygodowa
- Publicystyka
- Reportaż
- Romans i literatura obyczajowa
- Sensacja
- Thriller, Horror
- Wywiady i wspomnienia
-
Nauki przyrodnicze
-
Nauki społeczne
-
Popularnonaukowe i akademickie
-
Poradniki
-
Poradniki zawodowe i specjalistyczne
-
Prawo
-
Przewodniki i podróże
-
Psychologia
- Filozofie życiowe
- Komunikacja międzyludzka
- Mindfulness
- Ogólne
- Perswazja i NLP
- Psychologia akademicka
- Psychologia duszy i umysłu
- Psychologia pracy
- Relacje i związki
- Rodzicielstwo i psychologia dziecka
- Rozwiązywanie problemów
- Rozwój intelektualny
- Sekret
- Seksualność
- Uwodzenie
- Wygląd i wizerunek
- Życiowe filozofie
-
Religia
-
Sport, fitness, diety
-
Technika i mechanika
Kursy video
-
Bazy danych
-
Big Data
-
Biznes i ekonomia
-
Cyberbezpieczeństwo
-
Data Science
-
DevOps
-
Dla dzieci
-
Elektronika
-
Grafika/Wideo/CAX
-
Gry
-
Microsoft Office
-
Narzędzia programistyczne
-
Programowanie
-
Rozwój osobisty
-
Sieci komputerowe
-
Systemy operacyjne
-
Testowanie oprogramowania
-
Urządzenia mobilne
-
UX/UI
-
Web development
Podcasty
Szczegóły ebooka
Zaloguj się, jeśli jesteś zainteresowany treścią pozycji.
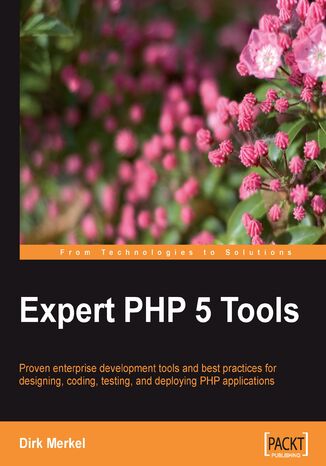
Expert PHP 5 Tools. Proven enterprise development tools and best practices for designing, coding, testing, and deploying PHP applications
Ebook
Even if you find writing PHP code easy, writing code that is efficient and easy to maintain and reuse is not so straightforward. Self-taught PHP developers and programmers transitioning from other languages often lack the knowledge to work with PHP on an enterprise level. They need to take their PHP development skills to that level by learning the skills and tools necessary to write maintainable and efficient code.This book will enable you to take your PHP development skills to an enterprise level by teaching you the skills and tools necessary to write maintainable and efficient code. You will learn how to perform activities such as unit testing, enforcing coding standards, automating deployment, and interactive debugging using tools created for PHP developers – all the information in one place. Your code will be more maintainable, efficient, and self-documented.From the design phase to actually deploying the application, you will learn concepts and apply them using the best-of-breed tools available in PHP.Experienced developers looking for expertise in PHP development will learn how to follow best practices within the world of PHP. The book contains many well-documented code samples and recipes that can be used as a starting point for producing quality code.Specifically, you will learn to design an application with UML, code it in Eclipse with PDT, document it with phpDocumentor, debug it interactively with Xdebug, test it by writing PHPUnit tests, manage source code in Subversion, speed up development and increase stability by using Zend Framework, pull everything together using continuous integration, and deploy the application automatically with Phing – all in one book. The author's experience in PHP development enables him to share insights on using enterprise tools, in a clear and friendly way.
- Expert PHP 5 Tools
- Table of Contents
- Expert PHP 5 Tools
- Credits
- About the Author
- About the Reviewers
- Preface
- What this book covers
- What you need for this book
- Who this book is for
- Conventions
- Reader feedback
- Customer support
- Downloading the example code for the book
- Errata
- Piracy
- Questions
- 1. Coding Style and Standards
- Coding standard considerations
- Pros
- Cons
- A PHP coding standard
- Formatting
- PHP tags
- Indenting
- Line length
- Line endings
- Spacing
- Statements
- Strings
- Arrays
- Control structures
- If-elseif-else statements
- Switch statements
- Class and method definitions
- Naming conventions
- Class names
- Property and variable names
- Constant names
- Method and function names
- Methodology
- Type hinting
- Separating object creation from initialization
- Class files
- Class names and directory structures
- Visibility and access
- Including source files
- Comments
- Inline documentation
- Formatting
- Coding standard adherence and verification
- PHP_CodeSniffer for automated checking
- Installing PHP_CodeSniffer
- Basic usage
- Slightly advanced usage
- Validating against your own coding standard
- Creating the directories
- The main coding standard class file
- Creating Sniffs
- Tokenization
- Writing our first sniff
- Extending existing sniffs
- Automated code checks
- PHP_CodeSniffer for automated checking
- Summary
- Coding standard considerations
- 2. Documentation with phpDocumentor
- Code-level documentation
- Levels of detail
- Introducing phpDocumentor
- Installing phpDocumentor
- DocBlocks
- Short and long descriptions
- Tags
- DocBlock templates
- Tutorials
- Naming conventions and how to reference tutorials
- DocBook syntax
- Documenting a project
- Documentation without DocBlocks
- Documentation with DocBlocks
- phpDocumentor options
- Command line reference
- Config files
- Browser-based interface
- Tag reference
- Standard tags
- @access
- @author
- @category
- @copyright
- @deprecated
- @example
- @filesource
- @global
- @ignore
- @internal
- @license
- @link
- @method
- @name
- @package
- @property
- @return
- @see
- @since
- @static
- @staticvar
- @subpackage
- @todo
- @tutorial
- @uses
- @var
- @version
- Inline tags
- {@Example}
- {@id}
- {@internal}}
- {@inheritdoc}
- {@link}
- {@source}
- {@toc}
- {@tutorial}
- Standard tags
- PHP4 elements
- Custom tags
- Summary
- Code-level documentation
- 3. The Eclipse Integrated Development Environment
- Why Eclipse?
- Introducing PDT
- Installing Eclipse
- Requirements
- Choosing a package
- Adding the PDT plugin
- Basic Eclipse concepts
- Workspace
- Views
- Perspectives
- A PDT sample project
- PDT features
- Editor
- Syntax highlighting
- Code assist
- Code folding
- Mark occurrences
- Override indicators
- Type, method, and resource navigation
- Inspection
- Projects and files
- PHP explorer
- Type hierarchy
- Debugging
- PDT preferences
- Appearance
- Code style
- Formatter
- Code templates
- Debug
- Installed debuggers
- Step filtering
- Workbench options
- Editor
- Code assist
- Code folding
- Hovers
- Mark occurrences
- Save actions
- Syntax coloring
- Task tags
- Typing
- New project layout
- PHP executables
- PHP interpreter
- PHP manual
- PHP servers
- Templates
- Other features
- PHP function reference
- Eclipse plugins
- Editor
- Zend Studio for Eclipse
- Support
- Refactoring
- Code generation
- PHPUnit testing
- PhpDocumentor support
- Zend Framework integration
- Zend server integration
- Summary
- 4. Source Code and Version Control
- Common use cases
- A brief history of source code control
- CVS
- Introducing Subversion
- Client installation
- Server configuration
- Apache with mod_dav_svn
- svnserve
- Apache with mod_dav_svn
- Subversion concepts
- Repository
- Tags
- Trunk
- Branches
- Working (Local) copy
- Merging
- Revisions and versions
- Updating
- Comparing
- History/Log
- Annotating code
- Reverting
- Committing
- Subversion command reference
- svn
- blame
- cat
- changelist
- checkout
- cleanup
- commit
- copy
- delete
- diff
- export
- help
- import
- info
- list
- lock
- log
- merge
- mergeinfo
- mkdir
- move
- propdel
- propedit
- propget
- proplist
- propset
- resolve
- resolved
- revert
- status
- switch
- unlock
- update
- svnadmin
- create
- dump
- svnlook
- svnserve
- svndumpfilter
- svnversion
- svn
- Creating a Subversion project
- Basic version control workflow
- A closer look at the repository
- Data store
- Layout
- Branching and merging
- What is a branch?
- Why branch?
- How to branch?
- Maintaining and merging a branch
- Branching workflow
- UI clients
- Eclipse plug-ins
- TortoiseSVN
- WebSVN
- Subversion conventions and best practices
- Customizing Subversion
- Hooks
- Enforcing coding standards with a pre-commit hook
- Notifying developers of commits with a post-commit hook
- Hooks
- Customizing Subversion
- Summary
- 5. Debugging
- First line of defense: syntax check
- Logging
- Configuration options
- Customizing and controlling config options: PhpIni
- PhpIni example
- Outputting debug information
- Functions
- echo(string $arg1 [, string $... ] / print(string $arg)
- var_dump(mixed $expression [, mixed $expression [, $... ]]) and print_r(mixed $expression [, bool $return= false ])
- highlight_string(string str [, bool return]) and highlight_file(string filename [, bool return])
- get_class([object object])
- get_object_vars(object object)
- get_class_methods(mixed class_name)
- get_class_vars(string class_name)
- debug_backtrace()
- debug_print_backtrace()
- exit([string status]) or exit (int status)
- Magic constants
- Writing our own debugging class
- Functional requirements
- DebugException
- Using DebugException
- DebugException: Pros and cons
- Functions
- Introducing Xdebug
- Installing Xdebug
- Configuring Xdebug
- Immediate benefits
- var_dump() improved
- var_dump() settings
- Errors and exceptions beautified
- Stack trace settings
- Protection from infinite recursion
- Remote debugging
- Remote server debug configuration
- Debugging client configuration
- Summary
- 6. PHP Frameworks
- Writing your own framework
- Evaluating and choosing frameworks
- Community and acceptance
- Feature road map
- Documentation
- Code quality
- Coding standards and compliance
- Project fit
- Easy to learn and adapt
- Open source
- Familiarity
- Their rules
- Popular PHP frameworks
- Zend
- CodeIgniter
- Symfony
- Yii
- Zend Framework application
- Feature list
- Application skeleton
- Important concepts
- Bootstrapping
- MVC
- Application structure detail
- Model: application/models/
- View: application/views/
- Controller: application/controllers/
- Configuration: application/configs/
- Library
- Public
- Tests
- Important concepts
- Enhancements
- Adding a layout
- Adding views
- Adding logging
- Adding a database
- Adding a model
- Adding a controller
- Putting it all together
- Summary
- 7. Testing
- Testing methods
- Black box
- White box
- Gray box
- Types of testing
- Unit testing
- Integration testing
- Regression testing
- System testing
- User acceptance testing
- Introducing PHPUnit
- Installing PHPUnit
- String search project
- BMH algorithm basics
- Implementing BMH
- Unit testing BoyerMooreStringSearch
- The test class
- Assertions
- Organization
- Our first unit test
- Extended test class features
- Fixtures
- Annotations
- Data providers
- Exceptions
- Automation: generating tests from classes
- Unimplemented and skipped tests
- Automation: generating classes from tests
- Test-driven development
- Enhancing our example with TDD
- Code coverage
- TestCase subclasses
- Summary
- Testing methods
- 8. Deploying Applications
- Goals and requirements
- Deploying your application
- Checking out and uploading files
- Displaying an under-maintenance message
- Upgrading and installing files
- Upgrading database schema and data
- Rotating log files and updating symbolic links
- Verifying the deployed application
- Automating deployment
- Phing
- Installing Phing
- Basic syntax and file structure
- Tasks
- Targets
- Properties and property files
- Types
- Filters
- Mappers
- The project tag
- Deploying a site
- Separating external dependencies
- Creating a build script
- Environment and properties
- Directory skeleton
- Subversion export and checkout
- Building files from templates
- Maintenance page
- Database backup
- Database migrations
- Going live
- Putting it all together
- Backing out
- Phing
- Summary
- 9. PHP Application Design with UML
- Meta-model versus notation versus our approach
- Levels of detail and purpose
- Round-trip and one-way tools
- Basic types of UML diagrams
- Diagrams
- Class diagrams
- Elements of a class
- Properties (Attributes)
- Methods (Operations)
- Static methods and properties
- A class diagram example
- Relationships
- Association
- Aggregation
- Composition
- Dependency
- Generalization
- Interfaces
- Example refactored
- Code generators
- Elements of a class
- Sequence diagrams
- Scope
- A sequence diagram of the network scanner
- Objects and lifelines
- Methods
- Creating and destroying object
- Loops and conditionals
- Synchronous versus asynchronous calls
- Use cases
- Use cases diagrams optional
- When to create use cases
- Example use case
- Background
- Typical scenario
- Example use case diagram
- Actors
- System boundary
- Use cases
- Relationships
- Class diagrams
- Summary
- 10. Continuous Integration
- The satellite systems
- Version control: Subversion
- Commit frequency
- Testing: PHPUnit
- Automation: Phing
- Coding style: PHP_CodeSniffer
- Documentation: PhpDocumentor
- Code coverage: Xdebug
- Version control: Subversion
- Environment setup considerations
- Do I need a dedicated CI server?
- Do I need a CI tool?
- CI tools
- XINC (Xinc Is Not CruiseControl)
- phpUnderControl
- Continuous integration with phpUnderControl
- Installation
- Installing CruiseControl
- Installing phpUnderControl
- Overlaying CruiseControl with phpUnderControl
- CruiseControl configuration
- Overview of the CI process and components
- CruiseControl and project layout
- Getting the project source
- Configuring the project: build.xml
- Configuring CruiseControl
- Advanced options
- Bootstrappers
- Publishers
- Running CruiseControl
- The overview page
- The tests page
- Metrics
- Coverage
- Documentation
- CodeSniffer
- PHPUnit PMD
- Replacing Ant with Phing
- Installation
- Summary
- The satellite systems
- Index
- Tytuł: Expert PHP 5 Tools. Proven enterprise development tools and best practices for designing, coding, testing, and deploying PHP applications
- Autor: Dirk Merkel
- Tytuł oryginału: Expert PHP 5 Tools. Proven enterprise development tools and best practices for designing, coding, testing, and deploying PHP applications
- ISBN: 9781847198396, 9781847198396
- Data wydania: 2010-03-30
- Format: Ebook
- Identyfikator pozycji: e_3cvt
- Wydawca: Packt Publishing