Categories
Ebooks
-
Business and economy
- Bitcoin
- Businesswoman
- Coaching
- Controlling
- E-business
- Economy
- Finances
- Stocks and investments
- Personal competence
- Computer in the office
- Communication and negotiation
- Small company
- Marketing
- Motivation
- Multimedia trainings
- Real estate
- Persuasion and NLP
- Taxes
- Social policy
- Guides
- Presentations
- Leadership
- Public Relation
- Reports, analyses
- Secret
- Social Media
- Sales
- Start-up
- Your career
- Management
- Project management
- Human Resources
-
For children
-
For youth
-
Education
-
Encyclopedias, dictionaries
-
E-press
- Architektura i wnętrza
- Health and Safety
- Biznes i Ekonomia
- Home and garden
- E-business
- Ekonomia i finanse
- Finances
- Personal finance
- Business
- Photography
- Computer science
- HR & Payroll
- For women
- Computers, Excel
- Accounts
- Culture and literature
- Scientific and academic
- Environmental protection
- Opinion-forming
- Education
- Taxes
- Travelling
- Psychology
- Religion
- Agriculture
- Book and press market
- Transport and Spedition
- Healthand beauty
-
History
-
Computer science
- Office applications
- Data bases
- Bioinformatics
- IT business
- CAD/CAM
- Digital Lifestyle
- DTP
- Electronics
- Digital photography
- Computer graphics
- Games
- Hacking
- Hardware
- IT w ekonomii
- Scientific software package
- School textbooks
- Computer basics
- Programming
- Mobile programming
- Internet servers
- Computer networks
- Start-up
- Operational systems
- Artificial intelligence
- Technology for children
- Webmastering
-
Other
-
Foreign languages
-
Culture and art
-
School reading books
-
Literature
- Antology
- Ballade
- Biographies and autobiographies
- For adults
- Dramas
- Diaries, memoirs, letters
- Epic, epopee
- Essay
- Fantasy and science fiction
- Feuilletons
- Work of fiction
- Humour and satire
- Other
- Classical
- Crime fiction
- Non-fiction
- Fiction
- Mity i legendy
- Nobelists
- Novellas
- Moral
- Okultyzm i magia
- Short stories
- Memoirs
- Travelling
- Narrative poetry
- Poetry
- Politics
- Popular science
- Novel
- Historical novel
- Prose
- Adventure
- Journalism, publicism
- Reportage novels
- Romans i literatura obyczajowa
- Sensational
- Thriller, Horror
- Interviews and memoirs
-
Natural sciences
-
Social sciences
-
School textbooks
-
Popular science and academic
- Archeology
- Bibliotekoznawstwo
- Cinema studies
- Philology
- Polish philology
- Philosophy
- Finanse i bankowość
- Geography
- Economy
- Trade. World economy
- History and archeology
- History of art and architecture
- Cultural studies
- Linguistics
- Literary studies
- Logistics
- Maths
- Medicine
- Humanities
- Pedagogy
- Educational aids
- Popular science
- Other
- Psychology
- Sociology
- Theatre studies
- Theology
- Economic theories and teachings
- Transport i spedycja
- Physical education
- Zarządzanie i marketing
-
Guides
-
Game guides
-
Professional and specialist guides
-
Law
- Health and Safety
- History
- Road Code. Driving license
- Law studies
- Healthcare
- General. Compendium of knowledge
- Academic textbooks
- Other
- Construction and local law
- Civil law
- Financial law
- Economic law
- Economic and trade law
- Criminal law
- Criminal law. Criminal offenses. Criminology
- International law
- International law
- Health care law
- Educational law
- Tax law
- Labor and social security law
- Public, constitutional and administrative law
- Family and Guardianship Code
- agricultural law
- Social law, labour law
- European Union law
- Industry
- Agricultural and environmental
- Dictionaries and encyclopedia
- Public procurement
- Management
-
Tourist guides and travel
- Africa
- Albums
- Southern America
- North and Central America
- Australia, New Zealand, Oceania
- Austria
- Asia
- Balkans
- Middle East
- Bulgary
- China
- Croatia
- The Czech Republic
- Denmark
- Egipt
- Estonia
- Europe
- France
- Mountains
- Greece
- Spain
- Holand
- Iceland
- Lithuania
- Latvia
- Mapy, Plany miast, Atlasy
- Mini travel guides
- Germany
- Norway
- Active travelling
- Poland
- Portugal
- Other
- Przewodniki po hotelach i restauracjach
- Russia
- Romania
- Slovakia
- Slovenia
- Switzerland
- Sweden
- World
- Turkey
- Ukraine
- Hungary
- Great Britain
- Italy
-
Psychology
- Philosophy of life
- Kompetencje psychospołeczne
- Interpersonal communication
- Mindfulness
- General
- Persuasion and NLP
- Academic psychology
- Psychology of soul and mind
- Work psychology
- Relacje i związki
- Parenting and children psychology
- Problem solving
- Intellectual growth
- Secret
- Sexapeal
- Seduction
- Appearance and image
- Philosophy of life
-
Religion
-
Sport, fitness, diets
-
Technology and mechanics
Audiobooks
-
Business and economy
- Bitcoin
- Businesswoman
- Coaching
- Controlling
- E-business
- Economy
- Finances
- Stocks and investments
- Personal competence
- Communication and negotiation
- Small company
- Marketing
- Motivation
- Real estate
- Persuasion and NLP
- Taxes
- Social policy
- Guides
- Presentations
- Leadership
- Public Relation
- Secret
- Social Media
- Sales
- Start-up
- Your career
- Management
- Project management
- Human Resources
-
For children
-
For youth
-
Education
-
Encyclopedias, dictionaries
-
E-press
-
History
-
Computer science
-
Other
-
Foreign languages
-
Culture and art
-
School reading books
-
Literature
- Antology
- Ballade
- Biographies and autobiographies
- For adults
- Dramas
- Diaries, memoirs, letters
- Epic, epopee
- Essay
- Fantasy and science fiction
- Feuilletons
- Work of fiction
- Humour and satire
- Other
- Classical
- Crime fiction
- Non-fiction
- Fiction
- Mity i legendy
- Nobelists
- Novellas
- Moral
- Okultyzm i magia
- Short stories
- Memoirs
- Travelling
- Poetry
- Politics
- Popular science
- Novel
- Historical novel
- Prose
- Adventure
- Journalism, publicism
- Reportage novels
- Romans i literatura obyczajowa
- Sensational
- Thriller, Horror
- Interviews and memoirs
-
Natural sciences
-
Social sciences
-
Popular science and academic
-
Guides
-
Professional and specialist guides
-
Law
-
Tourist guides and travel
-
Psychology
- Philosophy of life
- Interpersonal communication
- Mindfulness
- General
- Persuasion and NLP
- Academic psychology
- Psychology of soul and mind
- Work psychology
- Relacje i związki
- Parenting and children psychology
- Problem solving
- Intellectual growth
- Secret
- Sexapeal
- Seduction
- Appearance and image
- Philosophy of life
-
Religion
-
Sport, fitness, diets
-
Technology and mechanics
Videocourses
-
Data bases
-
Big Data
-
Biznes, ekonomia i marketing
-
Cybersecurity
-
Data Science
-
DevOps
-
For children
-
Electronics
-
Graphics/Video/CAX
-
Games
-
Microsoft Office
-
Development tools
-
Programming
-
Personal growth
-
Computer networks
-
Operational systems
-
Software testing
-
Mobile devices
-
UX/UI
-
Web development
-
Management
Podcasts
- Ebooks
- Programming
- Java
- Designing Hexagonal Architecture with Java. An architect's guide to building maintainable and change-tolerant applications with Java and Quarkus
E-book details
Log in, If you're interested in the contents of the item.
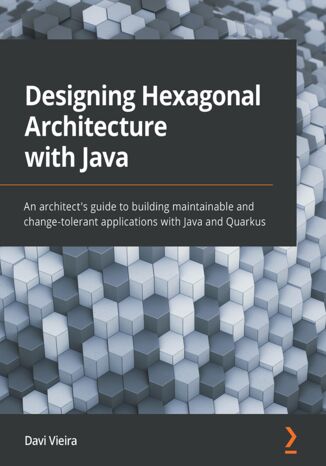
Designing Hexagonal Architecture with Java. An architect's guide to building maintainable and change-tolerant applications with Java and Quarkus
Ebook
Hexagonal architecture enhances developers' productivity by decoupling business code from technology code, making the software more change-tolerant, and allowing it to evolve and incorporate new technologies without the need for significant refactoring. By adhering to hexagonal principles, you can structure your software in a way that reduces the effort required to understand and maintain the code.
This book starts with an in-depth analysis of hexagonal architecture's building blocks, such as entities, use cases, ports, and adapters. You'll learn how to assemble business code in the Domain hexagon, create features by using ports and use cases in the Application hexagon, and make your software compatible with different technologies by employing adapters in the Framework hexagon. Moving on, you'll get your hands dirty developing a system based on a real-world scenario applying all the hexagonal architecture's building blocks. By creating a hexagonal system, you'll also understand how you can use Java modules to reinforce dependency inversion and ensure the isolation of each hexagon in the architecture. Finally, you'll get to grips with using Quarkus to turn your hexagonal application into a cloud-native system.
By the end of this hexagonal architecture book, you'll be able to bring order and sanity to the development of complex and long-lasting applications.
This book starts with an in-depth analysis of hexagonal architecture's building blocks, such as entities, use cases, ports, and adapters. You'll learn how to assemble business code in the Domain hexagon, create features by using ports and use cases in the Application hexagon, and make your software compatible with different technologies by employing adapters in the Framework hexagon. Moving on, you'll get your hands dirty developing a system based on a real-world scenario applying all the hexagonal architecture's building blocks. By creating a hexagonal system, you'll also understand how you can use Java modules to reinforce dependency inversion and ensure the isolation of each hexagon in the architecture. Finally, you'll get to grips with using Quarkus to turn your hexagonal application into a cloud-native system.
By the end of this hexagonal architecture book, you'll be able to bring order and sanity to the development of complex and long-lasting applications.
- 1. Why Hexagonal Architecture?
- 2. Wrapping Business Rules inside Domain Hexagon
- 3. Handling Behavior with Ports and Use Cases
- 4. Creating Adapters to Interact with the Outside World
- 5. Exploring the Nature of Driving and Driven Operations
- 6. Building the Domain Hexagon
- 7. Building the Application Hexagon
- 8. Building the Framework Hexagon
- 9. Applying Dependency Inversion with Java Modules
- 10. Adding Quarkus to a Modularized Hexagonal Application
- 11. Leveraging CDI Beans to Manage Ports and Use Cases
- 12. Using RESTEasy Reactive to Implement Input Adapters
- 13. Persisting Data with Output Adapters and Hibernate Reactive
- 14. Setting Up Dockerfile and Kubernetes Objects for Cloud Deployment
- 15. Good Design Practices for Your Hexagonal Application
- Title: Designing Hexagonal Architecture with Java. An architect's guide to building maintainable and change-tolerant applications with Java and Quarkus
- Author: Davi Vieira
- Original title: Designing Hexagonal Architecture with Java. An architect's guide to building maintainable and change-tolerant applications with Java and Quarkus
- ISBN: 9781801810296, 9781801810296
- Date of issue: 2022-01-07
- Format: Ebook
- Item ID: e_2t2z
- Publisher: Packt Publishing