Категорії
Електронні книги
-
Бізнес та економіка
- Біткойн
- Ділова жінка
- Коучинг
- Контроль
- Електронний бізнес
- Економіка
- Фінанси
- Фондова біржа та інвестиції
- Особисті компетенції
- Комп'ютер в офісі
- Комунікація та переговори
- Малий бізнес
- Маркетинг
- Мотивація
- Мультимедійне навчання
- Нерухомість
- Переконання та НЛП
- Податки
- Соціальна політика
- Порадники
- Презентації
- Лідерство
- Зв'язки з громадськістю
- Звіти, аналізи
- Секрет
- Соціальні засоби комунікації
- Продаж
- Стартап
- Ваша кар'єра
- Управління
- Управління проектами
- Людські ресурси (HR)
-
Для дітей
-
Для молоді
-
Освіта
-
Енциклопедії, словники
-
Електронна преса
- Architektura i wnętrza
- Безпека життєдіяльності
- Biznes i Ekonomia
- Будинок та сад
- Електронний бізнес
- Ekonomia i finanse
- Фінанси
- Особисті фінанси
- Бізнес
- Фотографія
- Інформатика
- Відділ кадрів та оплата праці
- Для жінок
- Комп'ютери, Excel
- Бухгалтерія
- Культура та література
- Наукові та академічні
- Охорона навколишнього середовища
- Впливові
- Освіта
- Податки
- Подорожі
- Психологія
- Релігія
- Сільське господарство
- Ринок книг і преси
- Транспорт та спедиція
- Здоров'я та краса
-
Історія
-
Інформатика
- Офісні застосунки
- Бази даних
- Біоінформатика
- Бізнес ІТ
- CAD/CAM
- Digital Lifestyle
- DTP
- Електроніка
- Цифрова фотографія
- Комп'ютерна графіка
- Ігри
- Хакування
- Hardware
- IT w ekonomii
- Наукові пакети
- Шкільні підручники
- Основи комп'ютера
- Програмування
- Мобільне програмування
- Інтернет-сервери
- Комп'ютерні мережі
- Стартап
- Операційні системи
- Штучний інтелект
- Технологія для дітей
- Вебмайстерність
-
Інше
-
Іноземні мови
-
Культура та мистецтво
-
Шкільні читанки
-
Література
- Антології
- Балада
- Біографії та автобіографії
- Для дорослих
- Драми
- Журнали, щоденники, листи
- Епос, епопея
- Нарис
- Наукова фантастика та фантастика
- Фельєтони
- Художня література
- Гумор, сатира
- Інше
- Класичний
- Кримінальний роман
- Нехудожня література
- Художня література
- Mity i legendy
- Лауреати Нобелівської премії
- Новели
- Побутовий роман
- Okultyzm i magia
- Оповідання
- Спогади
- Подорожі
- Оповідна поезія
- Поезія
- Політика
- Науково-популярна
- Роман
- Історичний роман
- Проза
- Пригодницька
- Журналістика
- Роман-репортаж
- Romans i literatura obyczajowa
- Сенсація
- Трилер, жах
- Інтерв'ю та спогади
-
Природничі науки
-
Соціальні науки
-
Шкільні підручники
-
Науково-популярна та академічна
- Археологія
- Bibliotekoznawstwo
- Кінознавство / Теорія кіно
- Філологія
- Польська філологія
- Філософія
- Finanse i bankowość
- Географія
- Економіка
- Торгівля. Світова економіка
- Історія та археологія
- Історія мистецтва і архітектури
- Культурологія
- Мовознавство
- літературні студії
- Логістика
- Математика
- Ліки
- Гуманітарні науки
- Педагогіка
- Навчальні засоби
- Науково-популярна
- Інше
- Психологія
- Соціологія
- Театральні студії
- Богослов’я
- Економічні теорії та науки
- Transport i spedycja
- Фізичне виховання
- Zarządzanie i marketing
-
Порадники
-
Ігрові посібники
-
Професійні та спеціальні порадники
-
Юридична
- Безпека життєдіяльності
- Історія
- Дорожній кодекс. Водійські права
- Юридичні науки
- Охорона здоров'я
- Загальне, компендіум
- Академічні підручники
- Інше
- Закон про будівництво і житло
- Цивільне право
- Фінансове право
- Господарське право
- Господарське та комерційне право
- Кримінальний закон
- Кримінальне право. Кримінальні злочини. Кримінологія
- Міжнародне право
- Міжнародне та іноземне право
- Закон про охорону здоров'я
- Закон про освіту
- Податкове право
- Трудове право та законодавство про соціальне забезпечення
- Громадське, конституційне та адміністративне право
- Кодекс про шлюб і сім'ю
- Аграрне право
- Соціальне право, трудове право
- Законодавство Євросоюзу
- Промисловість
- Сільське господарство та захист навколишнього середовища
- Словники та енциклопедії
- Державні закупівлі
- Управління
-
Путівники та подорожі
- Африка
- Альбоми
- Південна Америка
- Центральна та Північна Америка
- Австралія, Нова Зеландія, Океанія
- Австрія
- Азії
- Балкани
- Близький Схід
- Болгарія
- Китай
- Хорватія
- Чеська Республіка
- Данія
- Єгипет
- Естонія
- Європа
- Франція
- Гори
- Греція
- Іспанія
- Нідерланди
- Ісландія
- Литва
- Латвія
- Mapy, Plany miast, Atlasy
- Мініпутівники
- Німеччина
- Норвегія
- Активні подорожі
- Польща
- Португалія
- Інше
- Przewodniki po hotelach i restauracjach
- Росія
- Румунія
- Словаччина
- Словенія
- Швейцарія
- Швеція
- Світ
- Туреччина
- Україна
- Угорщина
- Велика Британія
- Італія
-
Психологія
- Філософія життя
- Kompetencje psychospołeczne
- Міжособистісне спілкування
- Mindfulness
- Загальне
- Переконання та НЛП
- Академічна психологія
- Психологія душі та розуму
- Психологія праці
- Relacje i związki
- Батьківство та дитяча психологія
- Вирішення проблем
- Інтелектуальний розвиток
- Секрет
- Сексуальність
- Спокушання
- Зовнішній вигляд та імідж
- Філософія життя
-
Релігія
-
Спорт, фітнес, дієти
-
Техніка і механіка
Аудіокниги
-
Бізнес та економіка
- Біткойн
- Ділова жінка
- Коучинг
- Контроль
- Електронний бізнес
- Економіка
- Фінанси
- Фондова біржа та інвестиції
- Особисті компетенції
- Комунікація та переговори
- Малий бізнес
- Маркетинг
- Мотивація
- Нерухомість
- Переконання та НЛП
- Податки
- Соціальна політика
- Порадники
- Презентації
- Лідерство
- Зв'язки з громадськістю
- Секрет
- Соціальні засоби комунікації
- Продаж
- Стартап
- Ваша кар'єра
- Управління
- Управління проектами
- Людські ресурси (HR)
-
Для дітей
-
Для молоді
-
Освіта
-
Енциклопедії, словники
-
Електронна преса
-
Історія
-
Інформатика
-
Інше
-
Іноземні мови
-
Культура та мистецтво
-
Шкільні читанки
-
Література
- Антології
- Балада
- Біографії та автобіографії
- Для дорослих
- Драми
- Журнали, щоденники, листи
- Епос, епопея
- Нарис
- Наукова фантастика та фантастика
- Фельєтони
- Художня література
- Гумор, сатира
- Інше
- Класичний
- Кримінальний роман
- Нехудожня література
- Художня література
- Mity i legendy
- Лауреати Нобелівської премії
- Новели
- Побутовий роман
- Okultyzm i magia
- Оповідання
- Спогади
- Подорожі
- Поезія
- Політика
- Науково-популярна
- Роман
- Історичний роман
- Проза
- Пригодницька
- Журналістика
- Роман-репортаж
- Romans i literatura obyczajowa
- Сенсація
- Трилер, жах
- Інтерв'ю та спогади
-
Природничі науки
-
Соціальні науки
-
Науково-популярна та академічна
-
Порадники
-
Професійні та спеціальні порадники
-
Юридична
-
Путівники та подорожі
-
Психологія
- Філософія життя
- Міжособистісне спілкування
- Mindfulness
- Загальне
- Переконання та НЛП
- Академічна психологія
- Психологія душі та розуму
- Психологія праці
- Relacje i związki
- Батьківство та дитяча психологія
- Вирішення проблем
- Інтелектуальний розвиток
- Секрет
- Сексуальність
- Спокушання
- Зовнішній вигляд та імідж
- Філософія життя
-
Релігія
-
Спорт, фітнес, дієти
-
Техніка і механіка
Відеокурси
-
Бази даних
-
Big Data
-
Biznes, ekonomia i marketing
-
Кібербезпека
-
Data Science
-
DevOps
-
Для дітей
-
Електроніка
-
Графіка / Відео / CAX
-
Ігри
-
Microsoft Office
-
Інструменти розробки
-
Програмування
-
Особистісний розвиток
-
Комп'ютерні мережі
-
Операційні системи
-
Тестування програмного забезпечення
-
Мобільні пристрої
-
UX/UI
-
Веброзробка, Web development
-
Управління
Подкасти
- Електронні книги
- Hardware
- Інше
- Oracle JRockit: The Definitive Guide. Understanding Adaptive Runtimes using JRockit R27/28
Деталі електронної книги
Увійти, Якщо вас цікавить зміст видання.
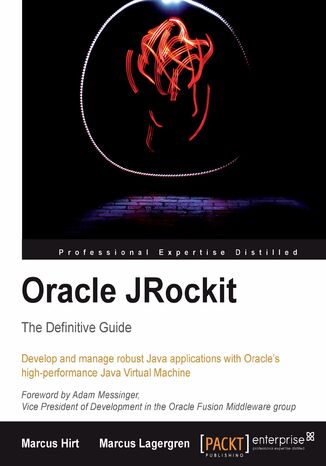
Oracle JRockit: The Definitive Guide. Understanding Adaptive Runtimes using JRockit R27/28
Eлектронна книга
Oracle JRockit is one of the industry’s highest performing Java Virtual Machines. Java developers are always on the lookout for better ways to analyze application behavior and gain performance. As we all know, this is not as easy as it looks. Welcome to JRockit: The Definitive Guide.This book helps you gain in-depth knowledge of Java from the JVM’s point of view. We will explain how to write code that works well with the JVM to gain performance and scalability. Starting with the inner workings of the JRockit JVM and finishing with a thorough walkthrough of the tools in the JRockit Mission Control suite, this book is for anyone who wants to know more about how the JVM executes your Java application and how to profile for better performance.
- Oracle JRockit The Definitive Guide
- Table of Contents
- Oracle JRockit
- Credits
- Foreword
- About the Authors
- Acknowledgement
- About the Reviewers
- Preface
- What this book covers
- What you need for this book
- Who this book is for
- Conventions
- Reader feedback
- Customer support
- Errata
- Piracy
- Questions
- 1. Getting Started
- Obtaining the JRockit JVM
- Migrating to JRockit
- Command-line options
- System properties
- Standardized options
- Non-standard options
- VM flags
- Changes in behavior
- Command-line options
- A note on JRockit versioning
- Getting help
- Summary
- 2. Adaptive Code Generation
- Platform independence
- The Java Virtual Machine
- Stack machine
- Bytecode format
- Operations and operands
- The constant pool
- Code generation strategies
- Pure bytecode interpretation
- Static compilation
- Total JIT compilation
- Mixed mode interpretation
- Adaptive code generation
- Determining "hotness"
- Invocation counters
- Software-based thread sampling
- Hardware-based sampling
- Optimizing a changing program
- Determining "hotness"
- Inside the JIT compiler
- Working with bytecode
- Bytecode obfuscation
- Bytecode "optimizers"
- Abstract syntax trees
- Where to optimize
- Working with bytecode
- The JRockit code pipeline
- Why JRockit has no bytecode interpreter
- Bootstrapping
- Runtime code generation
- Trampolines
- Code generation requests
- Optimization requests
- On-stack replacement
- Bookkeeping
- Object information for GC
- Source code and variable information
- Assumptions made about the generated code
- A walkthrough of method generation in JRockit
- The JRockit IR format
- Data flow
- Control flow
- A word about exceptions
- JIT compilation
- Generating HIR
- MIR
- LIR
- Register allocation
- Native code emission
- Generating optimized code
- A general overview
- How does the optimizer work?
- The JRockit IR format
- Controlling code generation in JRockit
- Command-line flags and directive files
- Command-line flags
- Logging
- Turning off optimizations
- Changing the number of code generation threads
- Directive files
- Command-line flags
- Command-line flags and directive files
- Summary
- 3. Adaptive Memory Management
- The concept of automatic memory management
- Adaptive memory management
- Advantages of automatic memory management
- Disadvantages of automatic memory management
- Fundamental heap management
- Allocating and releasing objects
- Fragmentation and compaction
- Garbage collection algorithms
- Reference counting
- Tracing techniques
- Mark and sweep
- Stop and copy
- Stopping the world
- Conservative versus exact collectors
- Livemaps
- Generational garbage collection
- Multi generation nurseries
- Write barriers
- Throughput versus low latency
- Optimizing for throughput
- Optimizing for low latency
- Garbage collection in JRockit
- Old collections
- Nursery collections
- Permanent generations
- Compaction
- Speeding it up and making it scale
- Thread local allocation
- Larger heaps
- 32-Bits and the 4-GB Barrier
- The 64-bit world
- Compressed references
- Cache friendliness
- Prefetching
- Data placement
- NUMA
- Large pages
- Adaptability
- Near-real-time garbage collection
- Hard and soft real-time
- JRockit Real Time
- Does the soft real-time approach work?
- How does it work?
- The Java memory API
- Finalizers
- References
- Weak references
- Soft references
- Phantom references
- Differences in JVM behavior
- Pitfalls and false optimizations
- Java is not C++
- Controlling JRockit memory management
- Basic switches
- Outputting GC data
- Set initial and maximum heap size
- Controlling what to optimize for
- Specifying a garbage collection strategy
- Compressed references
- Advanced switches
- Basic switches
- Summary
- The concept of automatic memory management
- 4. Threads and Synchronization
- Fundamental concepts
- Hard to debug
- Difficult to optimize
- Latency analysis
- Java API
- The synchronized keyword
- The java.lang.Thread class
- The java.util.concurrent package
- Semaphores
- The volatile keyword
- Implementing threads and synchronization in Java
- The Java Memory Model
- Early problems and ambiguities
- Immutability
- JSR-133
- Early problems and ambiguities
- Implementing synchronization
- Primitives
- Locks
- Thin locks
- Fat locks
- A word on fairness
- The lock word in JRockit
- The Java bytecode implementation
- Lock pairing
- Implementing threads
- Green threads
- N x M threads
- OS threads
- Thread pooling
- Green threads
- The Java Memory Model
- Optimizing threads and synchronization
- Lock inflation and lock deflation
- Recursive locking
- Lock fusion
- Lazy unlocking
- Implementation
- Object banning
- Class banning
- Results
- Pitfalls and false optimizations
- Thread.stop, Thread.resume and Thread.suspend
- Double checked locking
- JRockit flags
- Examining locks and lazy unlocking
- Lock details from -Xverbose:locks
- Controlling lazy unlocking withXX:UseLazyUnlocking
- Using SIGQUIT or Ctrl-Break for Stack Traces
- Lock profiling
- Enabling lock profiling with -XX:UseLockProfiling
- JRCMD
- Enabling lock profiling with -XX:UseLockProfiling
- Setting thread stack size using -Xss
- Controlling lock heuristics
- Examining locks and lazy unlocking
- Summary
- Fundamental concepts
- 5. Benchmarking and Tuning
- Reasons for benchmarking
- Performance goals
- Performance regression testing
- Easier problem domains to optimize
- Commercial success
- What to think of when creating a benchmark
- Measuring outside the system
- Measuring several times
- Micro benchmarks
- Micro benchmarks and on-stack replacement
- Micro benchmarks and startup time
- Give the benchmark a chance to warm-up
- Deciding what to measure
- Throughput
- Throughput with response time and latency
- Scalability
- Power consumption
- Other issues
- Industry-standard benchmarks
- The SPEC benchmarks
- The SPECjvm suite
- The SPECjAppServer / SPECjEnterprise2010 suite
- The SPECjbb suite
- SipStone
- The DaCapo benchmarks
- Real world applications
- The SPEC benchmarks
- The dangers of benchmarking
- Tuning
- Out of the box behavior
- What to tune for
- Tuning memory management
- Heap sizes
- The GC algorithm
- Compaction
- Tweaking System.gc
- Nursery size
- GC strategies
- Thread local area size and large objects
- Number of GC threads
- NUMA and CPU affinity
- Tuning code generation
- Call profiling
- Changing the number of optimization threads
- Turning off code optimizations
- Tuning locks and threads
- Lazy unlocking
- Enforcing thread priorities
- Thresholds for inflation and deflation
- Generic tuning
- Compressed references
- Large pages
- Tuning memory management
- Common bottlenecks and how to avoid them
- The XXaggressive flag
- Too many finalizers
- Too many reference objects
- Object pooling
- Bad algorithms and data structures
- Classic textbook issues
- Unwanted intrinsic properties
- Misuse of System.gc
- Too many threads
- One contended lock is the global bottleneck
- Unnecessary exceptions
- Large objects
- Native memory versus heap memory
- Wait/notify and fat locks
- Wrong heap size
- Too much live data
- Java is not a silver bullet
- Summary
- Reasons for benchmarking
- 6. JRockit Mission Control
- Background
- Sampling-based profiling versus exact profiling
- A different animal to different people
- Mission Control overview
- Mission Control server-side components
- Mission Control client-side components
- Terminology
- Running the standalone version of Mission Control
- Running JRockit Mission Control inside Eclipse
- Starting JRockit for remote management
- The JRockit Discovery Protocol
- Running in a secure environment
- Troubleshooting connections
- Hostname resolution issues
- The Experimental Update Site
- Debugging JRockit Mission Control
- Summary
- Background
- 7. The Management Console
- A JMX Management Console
- Using the console
- General
- The Overview
- MBeans
- MBean Browser
- Triggers
- Runtime
- System
- Memory
- Threads
- Advanced
- Method Profiler
- Exception Count
- Diagnostic Commands
- Other
- JConsole
- General
- Extending the JRockit Mission Control Console
- Summary
- 8. The Runtime Analyzer
- The need for feedback
- Recording
- Analyzing JRA recordings
- General
- Overview
- Recording
- System
- Memory
- Overview
- GCs
- GC Statistics
- Allocation
- Heap Contents
- Object Statistics
- Code
- Overview
- Hot Methods
- Optimizations
- Thread/Locks
- Overview
- Threads
- Java Locks
- JVM Locks
- Thread Dumps
- Latency
- Overview
- Log
- Graph
- Threads
- Traces
- Histogram
- Using the Operative Set
- General
- Troubleshooting
- Summary
- The need for feedback
- 9. The Flight Recorder
- The evolved Runtime Analyzer
- A word on events
- The recording engine
- Startup options
- Starting time-limited recordings
- Flight Recorder in JRockit Mission Control
- Advanced Flight Recorder Wizard concepts
- Differences to JRA
- The range selector
- The Operative Set
- The relational key
- Whats in a Latency?
- Exception profiling
- Memory
- Adding custom events
- Extending the Flight Recorder client
- Summary
- The evolved Runtime Analyzer
- 10. The Memory Leak Detector
- A Java memory leak
- Memory leaks in static languages
- Memory leaks in garbage collected languages
- Detecting a Java memory leak
- Memleak technology
- Tracking down the leak
- A look at classloader-related information
- Interactive memory leak hunting
- The general purpose heap analyzer
- Allocation traces
- Troubleshooting Memleak
- Summary
- A Java memory leak
- 11. JRCMD
- Introduction
- Overriding SIGQUIT
- Special commands
- Limitations of JRCMD
- JRCMD command reference
- check_flightrecording (R28)
- checkjrarecording (R27)
- command_line
- dump_flightrecording (R28)
- heap_diagnostics (R28)
- hprofdump (R28)
- kill_management_server
- list_vmflags (R28)
- lockprofile_print
- lockprofile_reset
- memleakserver
- oom_diagnostics (R27)
- print_class_summary
- print_codegen_list
- print_memusage (R27)
- print_memusage (R28)
- print_object_summary
- print_properties
- print_threads
- print_utf8pool
- print_vm_state
- run_optfile (R27)
- run_optfile (R28)
- runfinalization
- runsystemgc
- set_vmflag (R28)
- start_flightrecording (R28)
- start_management_server
- startjrarecording (R27)
- stop_flightrecording (R28)
- timestamp
- verbosity
- version
- Summary
- 12. Using the JRockit Management APIs
- JMAPI
- JMAPI examples
- JMXMAPI
- The JRockit internal performance counters
- An examplebuilding a remote version of JRCMD
- Summary
- JMAPI
- 13. JRockit Virtual Edition
- Introduction to virtualization
- Full virtualization
- Paravirtualization
- Other virtualization keywords
- Hypervisors
- Hosted hypervisors
- Native hypervisors
- Hypervisors in the market
- Advantages of virtualization
- Disadvantages of virtualization
- Virtualizing Java
- Introducing JRockit Virtual Edition
- The JRockit VE kernel
- The virtual machine image concept and management frameworks
- Benefits of JRockit VE
- Performance and better resource utilization
- Getting rid of "Triple virtualization"
- Memory footprint
- Manageability
- Simplicity and security
- Performance and better resource utilization
- Constraints and limitations of JRockit VE
- Introducing JRockit Virtual Edition
- A look aheadcan virtual be faster than real?
- Quality of hot code samples
- Adaptive heap resizing
- Inter-thread page protection
- Improved garbage collection
- Concurrent compaction
- Summary
- Introduction to virtualization
- A. Bibliography
- B. Glossary
- Abstract syntax tree
- Access file
- Adaptive code generation
- Adaptive memory management
- Agent
- Ahead-of-time compilation
- Allocation profiling
- AST
- Atomic instructions
- Automatic memory management
- Balloon driver
- Basic block
- Benchmark driver
- Biased locking
- Bytecode
- Bytecode interpretation
- Call profiling
- Card
- Card Table
- CAS
- Class block
- Class garbage collection
- Client-side template
- Cloud
- Code generation queue
- Color
- Compaction
- Compare and swap
- Compressed references
- Concurrent garbage collection
- Conservative garbage collection
- Constant pool
- Continuous JRA
- Control flow graph
- CPU profiling
- Critical section
- Dead code
- Deadlock
- Deadlock detection
- Design mode
- Deterministic garbage collection
- Diagnostic command
- Double-checked locking
- Driver
- Editor
- Escape analysis
- Event
- Event attribute
- Event field
- Event settings
- Event type
- Exact garbage collection
- Exact profiling
- Extension point
- Fairness
- Fat lock
- Fragmentation
- Free list
- Full virtualization
- GC heuristic
- GC pause ratio
- GC strategy
- Generation
- Generational garbage collection
- Graph coloring
- Graph fusion
- Green threads
- Guard page
- Guest
- Hard real-time
- Hardware prefetching
- Heap
- HIR
- Hosted hypervisor
- Hypervisor
- Inlining
- Intermediate representation
- Internal pointer
- IR
- Invocation counters
- Java bytecode
- Java Memory Model
- JFR
- JIT compilation
- JMAPI
- JMX
- JMXMAPI
- JRA
- JRCMD
- JRMC
- JRockit
- JRockit Flight Recorder
- JRockit Memory Leak Detector
- JRockit Mission Control
- JRockit Runtime Analyzer
- JSR
- JSR-133
- JSR-174
- JSR-292
- JVM Browser
- Keystore
- Lane
- Large pages
- Latency
- Latency threshold
- Lazy unlocking
- LIR
- Livelock
- Livemap
- Live object
- Live set
- Live Set + Fragmentation
- Lock deflation
- Lock fusion
- Lock inflation
- Lock pairing
- Lock token
- Lock word
- Mark and sweep
- Master password
- MBean
- MBean server
- MD5
- Memleak
- Memory Model
- Method garbage collection
- Micro benchmark
- MIR
- Mixed mode interpretation
- Monitor
- Name mangling
- Native code
- Native hypervisor
- Native memory
- Native threads
- Non-contiguous heaps
- NxM threads
- NUMA
- Nursery
- Obfuscation
- Object header
- Object pooling
- Old space
- On-stack replacement
- Operative set
- Optimization queue
- Out of the box behavior
- Overprovisioning
- OS threads
- Page protection
- Parallel garbage collection
- Paravirtualization
- Password file
- PDE
- Perspective
- Phantom References
- Prefetching
- Producer
- Promotion
- RCP
- Read barrier
- Real-time
- Recording agent
- Recording engine
- Recursive lock
- Reference compression
- Reference counting
- Reference decompression
- Register allocation
- Relational key
- Rich client platform
- Role
- Rollforwarding
- Root set
- Run mode
- Safepoint
- Samples
- Sample-based profiling
- Semaphore
- Server-side template
- Soft real-time
- Soft references
- Software prefetching
- Spilling
- Spinlock
- SSA form
- Static compilation
- Stop and copy
- Stopping the world
- Strong references
- SWT
- Synthetic attribute
- Tab group
- Tab group toolbar
- Thin lock
- Thread local allocation
- Thread local area
- Thread local heap
- Thread pooling
- Thread sampling
- Throughput
- TLA
- Tracing garbage collection
- Trampoline
- Trigger action
- Trigger condition
- Trigger constraint
- Trigger rule
- Truststore
- Virtualization
- Virtual machine image
- Volatile fields
- Warm up round
- Weak reference
- Write barrier
- Young space
- Index
- Назва: Oracle JRockit: The Definitive Guide. Understanding Adaptive Runtimes using JRockit R27/28
- Автор: Marcus Hirt, Marcus Lagergren
- Оригінальна назва: Oracle JRockit: The Definitive Guide. Understanding Adaptive Runtimes using JRockit R27/28
- ISBN: 9781847198075, 9781847198075
- Дата видання: 2010-06-01
- Формат: Eлектронна книга
- Ідентифікатор видання: e_3awv
- Видавець: Packt Publishing