Categories
Ebooks
-
Business and economy
- Bitcoin
- Businesswoman
- Coaching
- Controlling
- E-business
- Economy
- Finances
- Stocks and investments
- Personal competence
- Computer in the office
- Communication and negotiation
- Small company
- Marketing
- Motivation
- Multimedia trainings
- Real estate
- Persuasion and NLP
- Taxes
- Social policy
- Guides
- Presentations
- Leadership
- Public Relation
- Reports, analyses
- Secret
- Social Media
- Sales
- Start-up
- Your career
- Management
- Project management
- Human Resources
-
For children
-
For youth
-
Education
-
Encyclopedias, dictionaries
-
E-press
- Architektura i wnętrza
- Biznes i Ekonomia
- Home and garden
- E-business
- Finances
- Personal finance
- Business
- Photography
- Computer science
- HR & Payroll
- Computers, Excel
- Accounts
- Culture and literature
- Scientific and academic
- Environmental protection
- Opinion-forming
- Education
- Taxes
- Travelling
- Psychology
- Religion
- Agriculture
- Book and press market
- Transport and Spedition
- Healthand beauty
-
History
-
Computer science
- Office applications
- Data bases
- Bioinformatics
- IT business
- CAD/CAM
- Digital Lifestyle
- DTP
- Electronics
- Digital photography
- Computer graphics
- Games
- Hacking
- Hardware
- IT w ekonomii
- Scientific software package
- School textbooks
- Computer basics
- Programming
- Mobile programming
- Internet servers
- Computer networks
- Start-up
- Operational systems
- Artificial intelligence
- Technology for children
- Webmastering
-
Other
-
Foreign languages
-
Culture and art
-
School reading books
-
Literature
- Antology
- Ballade
- Biographies and autobiographies
- For adults
- Dramas
- Diaries, memoirs, letters
- Epic, epopee
- Essay
- Fantasy and science fiction
- Feuilletons
- Work of fiction
- Humour and satire
- Other
- Classical
- Crime fiction
- Non-fiction
- Fiction
- Mity i legendy
- Nobelists
- Novellas
- Moral
- Okultyzm i magia
- Short stories
- Memoirs
- Travelling
- Narrative poetry
- Poetry
- Politics
- Popular science
- Novel
- Historical novel
- Prose
- Adventure
- Journalism, publicism
- Reportage novels
- Romans i literatura obyczajowa
- Sensational
- Thriller, Horror
- Interviews and memoirs
-
Natural sciences
-
Social sciences
-
School textbooks
-
Popular science and academic
- Archeology
- Bibliotekoznawstwo
- Cinema studies
- Philology
- Polish philology
- Philosophy
- Finanse i bankowość
- Geography
- Economy
- Trade. World economy
- History and archeology
- History of art and architecture
- Cultural studies
- Linguistics
- Literary studies
- Logistics
- Maths
- Medicine
- Humanities
- Pedagogy
- Educational aids
- Popular science
- Other
- Psychology
- Sociology
- Theatre studies
- Theology
- Economic theories and teachings
- Transport i spedycja
- Physical education
- Zarządzanie i marketing
-
Guides
-
Game guides
-
Professional and specialist guides
-
Law
- Health and Safety
- History
- Road Code. Driving license
- Law studies
- Healthcare
- General. Compendium of knowledge
- Academic textbooks
- Other
- Construction and local law
- Civil law
- Financial law
- Economic law
- Economic and trade law
- Criminal law
- Criminal law. Criminal offenses. Criminology
- International law
- International law
- Health care law
- Educational law
- Tax law
- Labor and social security law
- Public, constitutional and administrative law
- Family and Guardianship Code
- agricultural law
- Social law, labour law
- European Union law
- Industry
- Agricultural and environmental
- Dictionaries and encyclopedia
- Public procurement
- Management
-
Tourist guides and travel
- Africa
- Albums
- Southern America
- North and Central America
- Australia, New Zealand, Oceania
- Austria
- Asia
- Balkans
- Middle East
- Bulgary
- China
- Croatia
- The Czech Republic
- Denmark
- Egipt
- Estonia
- Europe
- France
- Mountains
- Greece
- Spain
- Holand
- Iceland
- Lithuania
- Latvia
- Mapy, Plany miast, Atlasy
- Mini travel guides
- Germany
- Norway
- Active travelling
- Poland
- Portugal
- Other
- Russia
- Romania
- Slovakia
- Slovenia
- Switzerland
- Sweden
- World
- Turkey
- Ukraine
- Hungary
- Great Britain
- Italy
-
Psychology
- Philosophy of life
- Kompetencje psychospołeczne
- Interpersonal communication
- Mindfulness
- General
- Persuasion and NLP
- Academic psychology
- Psychology of soul and mind
- Work psychology
- Relacje i związki
- Parenting and children psychology
- Problem solving
- Intellectual growth
- Secret
- Sexapeal
- Seduction
- Appearance and image
- Philosophy of life
-
Religion
-
Sport, fitness, diets
-
Technology and mechanics
Audiobooks
-
Business and economy
- Bitcoin
- Businesswoman
- Coaching
- Controlling
- E-business
- Economy
- Finances
- Stocks and investments
- Personal competence
- Communication and negotiation
- Small company
- Marketing
- Motivation
- Real estate
- Persuasion and NLP
- Taxes
- Guides
- Presentations
- Leadership
- Public Relation
- Secret
- Social Media
- Sales
- Start-up
- Your career
- Management
- Project management
- Human Resources
-
For children
-
For youth
-
Education
-
Encyclopedias, dictionaries
-
History
-
Computer science
-
Other
-
Foreign languages
-
Culture and art
-
School reading books
-
Literature
- Antology
- Ballade
- Biographies and autobiographies
- For adults
- Dramas
- Diaries, memoirs, letters
- Epic, epopee
- Essay
- Fantasy and science fiction
- Feuilletons
- Work of fiction
- Humour and satire
- Other
- Classical
- Crime fiction
- Non-fiction
- Fiction
- Mity i legendy
- Nobelists
- Novellas
- Moral
- Okultyzm i magia
- Short stories
- Memoirs
- Travelling
- Poetry
- Politics
- Popular science
- Novel
- Historical novel
- Prose
- Adventure
- Journalism, publicism
- Reportage novels
- Romans i literatura obyczajowa
- Sensational
- Thriller, Horror
- Interviews and memoirs
-
Natural sciences
-
Social sciences
-
Popular science and academic
-
Guides
-
Professional and specialist guides
-
Law
-
Tourist guides and travel
-
Psychology
- Philosophy of life
- Interpersonal communication
- Mindfulness
- General
- Persuasion and NLP
- Academic psychology
- Psychology of soul and mind
- Work psychology
- Relacje i związki
- Parenting and children psychology
- Problem solving
- Intellectual growth
- Secret
- Sexapeal
- Seduction
- Appearance and image
- Philosophy of life
-
Religion
-
Sport, fitness, diets
-
Technology and mechanics
Videocourses
-
Data bases
-
Big Data
-
Biznes, ekonomia i marketing
-
Cybersecurity
-
Data Science
-
DevOps
-
For children
-
Electronics
-
Graphics/Video/CAX
-
Games
-
Microsoft Office
-
Development tools
-
Programming
-
Personal growth
-
Computer networks
-
Operational systems
-
Software testing
-
Mobile devices
-
UX/UI
-
Web development
-
Management
Podcasts
E-book details
Log in, If you're interested in the contents of the item.
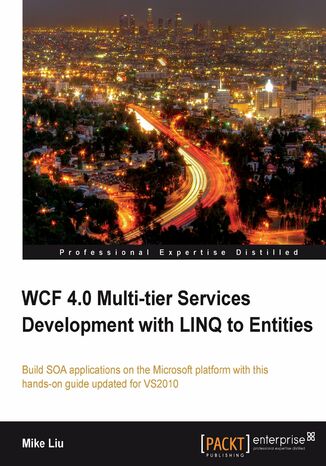
WCF 4.0 Multi-tier Services Development with LINQ to Entities. Build SOA applications on the Microsoft platform with this hands-on guide updated for VS2010
Ebook
WCF is the Microsoft model for building services, whereas LINQ to Entities is the Microsoft ORM for accessing underlying data storage. Want to learn both? You would normally have to dig through huge reference tomes—so wouldn't you agree that a simple-to-follow practical tutorial on WCF and LINQ to Entities is the way to get ahead?This book is the quickest and easiest way to learn WCF and LINQ to Entities in Visual Studio 2010. WCF and LINQ to Entities are both powerful yet complex technologies from Microsoft—but you will be surprised at how easily this book will get you get up and running with them.Mastery of these two topics will quickly enable you to create Service-Oriented applications, and allow you to take your first steps into the world of Service Oriented Architecture without becoming overwhelmed.Through this book, you will learn what's going on behind the scenes with WCF, and dive into the basic yet most useful techniques for LINQ to Entities. You will develop three real-world multi-tiered WCF services from beginning to end, with LINQ to Entities being used in the data access layer of the services. Various clients including windows console applications, the WCF Test Client, Windows Form applications and WPF applications will be created to test these WCF services. By the end of this book, you will be 100% confident that you know WCF and LINQ to Entities, not only in theory, but with sound real-world experience.
- WCF 4.0 Multi-tier Services Development with LINQ to Entities
- WCF 4.0 Multi-tier Services Development with LINQ to Entities
- Credits
- About the Author
- About the Reviewers
- Preface
- What this book covers
- What you need for this book
- Who this book is for
- Conventions
- Reader feedback
- Customer support
- Errata
- Piracy
- Questions
- 1. Introducing Web Services and Windows Communication Foundation
- What is SOA?
- Web services
- What is a web service?
- Web service WSDL
- Web service proxy
- SOAP
- Web services: standards and specifications
- WS-I Profiles
- WS-Addressing
- WS-Security
- WS-ReliableMessaging
- WS-Coordination and WS-Transaction
- WCF: Windows Communication Foundation
- What is WCF?
- Why is WCF used for SOA?
- WCF architecture
- Basic WCF conceptsWCF ABCs
- Address
- Binding
- Contract
- Service contract
- Operation contract
- Message contract
- Data contract
- Fault contract
- Endpoint
- Behavior
- Hosting
- Self hosting
- Windows services hosting
- IIS hosting
- Windows Activation Services hosting
- Channels
- Metadata
- WCF production and development environments
- Summary
- 2. Implementing a Basic HelloWorld WCF Service
- Creating the HelloWorld solution and project
- Creating the HelloWorldService service contract interface
- Implementing the HelloWorldService service contract
- Hosting the WCF service in ASP.NET Development Server
- Creating the host application
- Testing the host application
- ASP.NET Development Server
- Adding an SVC file to the host application
- Modifying the web.config file
- Starting the host application
- Creating a client to consume the WCF service
- Creating the client application project
- Generating the proxy and configuration files
- Customizing the client application
- Running the client application
- Setting the service application to AutoStart
- Summary
- 3. Hosting and Debugging the HelloWorld WCF Service
- Hosting the HelloWorld WCF service
- Hosting the service in a managed application
- Hosting the service in a console application
- Consuming the service hosted in a console application
- Hosting the service in a Windows service
- Hosting the service in Internet Information Server
- Preparing the folders and files
- Turn on Internet Information Services
- Creating the IIS application
- Starting the WCF service in IIS
- Testing the WCF service hosted in IIS
- Other WCF service hosting options
- Hosting the service in a managed application
- Debugging the HelloWorld WCF service
- Debugging from the client application
- Starting the debugging process
- Debugging on the client application
- Attaching to ASP.NET Development Server
- Stepping into the WCF service
- Debugging only the WCF service
- Starting the WCF Service in debugging mode
- Starting the client application in non-debugging mode
- Starting the WCF service and client applications in debugging mode
- Attaching to a WCF service process
- Running the WCF service and client applications in non-debugging mode
- Debugging the WCF service hosted in IIS
- Just-In-Time debugger
- Debugging from the client application
- Summary
- Hosting the HelloWorld WCF service
- 4. Implementing a WCF Service in the Real World
- Why layer a service?
- Creating a new solution and project using WCF templates
- Using the C# WCF service library template
- Using the C# WCF service application template
- Creating the service interface layer
- Creating the service interfaces
- Creating the data contracts
- Implementing the service contracts
- Modifying the app.config file
- Testing the service using WCF Test Client
- Testing the service using our own client
- Adding a business logic layer
- Adding the product entity project
- Adding the business logic project
- Calling the business logic layer from the service interface layer
- Testing the WCF service with a business logic layer
- Summary
- 5. Adding Database Support and Exception Handling to the RealNorthwind WCF Service
- Adding a data access layer
- Creating the data access layer project
- Calling the data access layer from the business logic layer
- Preparing the database
- Adding the connection string to the configuration file
- Querying the database (GetProduct)
- Testing the GetProduct method
- Updating the database (UpdateProduct)
- Adding error handling to the service
- Adding a fault contract
- Throwing a fault exception
- Updating the client program to catch the fault exception
- Testing the fault exception
- Summary
- Adding a data access layer
- 6. LINQLanguage Integrated Query
- What is LINQ
- Creating the test solution and project
- New data type var
- Automatic properties
- Object initializer
- Collection initializer
- Anonymous types
- Extension methods
- Lambda expressions
- Built-in LINQ extension methods and method syntax
- LINQ query syntax and query expression
- Built-in LINQ operators
- Summary
- 7. LINQ to Entities: Basic Concepts and Features
- ORMObject-Relational Mapping
- Entity Framework
- LINQ to Entities
- Comparing LINQ to Entities with LINQ to Objects
- LINQ to SQL
- Comparing LINQ to SQL with LINQ to Entities
- Creating a LINQ to Entities test application
- Creating the Data Model
- Adding a LINQ to Entities item to the project
- Generated LINQ to Entities classes
- Querying and updating the database with a table
- Querying records
- Updating records
- Inserting records
- Deleting records
- Running the program
- View Generated SQL statements
- View SQL statements using ToTraceString
- View SQL statements using Profiler
- Deferred execution
- Checking deferred execution with SQL profiler
- Deferred execution for singleton methods
- Deferred execution for singleton methods within sequence expressions
- Deferred (lazy) loading versus eager loading
- Lazy loading by default
- Eager loading the with Include method
- Joining two tables
- Querying a view
- Summary
- 8. LINQ to Entities: Advanced Concepts and Features
- Calling a stored procedure
- Mapping a stored procedure to a new entity class
- Modeling a stored procedure
- Querying a stored procedure
- Mapping a stored procedure to an existing entity class
- Mapping a stored procedure to a new entity class
- Compiled query
- Direct SQL
- Dynamic query
- Dynamic query with expressions
- Dynamic query with parameters
- Inheritance
- LINQ to Entities Table per Hierarchy inheritance
- Modeling the BaseCustomer and USACustomer entities
- Modeling the UKCustomer entity
- Generated classes with TPH inheritance
- Testing the TPH inheritance
- LINQ to Entities Table per Type inheritance
- Preparing database tables
- Modeling USACustomer1 and UKCustomer1 entities
- Generated classes with TPT inheritance
- Testing the TPT inheritance
- LINQ to Entities Table per Hierarchy inheritance
- Handling simultaneous (concurrent) updates
- Detecting conflicts using a data column
- Explaining the Concurrency Mode property
- Adding another Entity Data Model
- Writing the test code
- Testing the conflicts
- Turning on concurrency control
- Detecting conflicts using a version column
- Adding a version column
- Modeling the Products table with a version column
- Writing the test code
- Testing the conflicts
- Detecting conflicts using a data column
- Transaction support
- Implicit transactions
- Explicit transactions
- Adding validations to entity classes
- Debugging LINQ to Entities programs
- Summary
- Calling a stored procedure
- 9. Applying LINQ to Entities to a WCF Service
- Creating the LINQNorthwind solution
- Modeling the Northwind database
- Copying the connection string to the service layer
- Using LINQ to Entities in the data access layer
- Modifying GetProduct in the data access layer
- Modifying UpdateProduct in the data access layer
- Testing LINQ to Entities with the WCF Test Client
- Adding concurrency support
- Turning on RowVersion concurrency mode
- Modifying the ProductEntity class
- Modifying the ProductDAO class
- Modifying the GetProduct method
- Modifying UpdateProduct method
- Modifying the business logic layer classes
- Modifying the service interface layer classes
- Testing concurrency with WCF Test Client
- Testing concurrency with our own client
- Creating the test client
- Implementing the GetProduct functionality
- Implementing the UpdateProduct functionality
- Testing the GetProduct and UpdateProduct operations
- Testing concurrent update manually
- Testing concurrent update automatically
- Creating the test client
- Summary
- 10. Distributed Transaction Support of WCF
- Creating the DistNorthwind solution
- Hosting the WCF service in IIS
- Testing the transaction behavior of the WCF service
- Creating a client to call the WCF service sequentially
- Testing the sequential calls to the WCF service
- Wrapping the WCF service calls in one transaction scope
- Testing multiple database support of the WCF service
- Creating a new WCF service
- Calling the new WCF service in the client application
- Testing the WCF service with two databases
- Enabling distributed transaction support
- Enabling transaction flow in service binding
- Enabling transaction flow on the service hosting application
- Modifying the service operation contract to allow a transaction flow
- Modifying the service operation implementation to require a transaction scope
- Enabling transaction flow in service binding
- Understanding the distributed transaction support of a WCF service
- Testing the distributed transaction support of the WCF service
- Configuring the Distributed Transaction Coordinator
- Configuring the firewall
- Propagating a transaction from the client to the WCF service
- Testing distributed transaction support with one database
- Testing distributed transaction support with two databases
- Summary
- Title: WCF 4.0 Multi-tier Services Development with LINQ to Entities. Build SOA applications on the Microsoft platform with this hands-on guide updated for VS2010
- Author: Mike Liu, Hongcheng Lui
- Original title: WCF 4.0 Multi-tier Services Development with LINQ to Entities. Build SOA applications on the Microsoft platform with this hands-on guide updated for VS2010
- ISBN: 9781849681155, 9781849681155
- Date of issue: 2010-06-21
- Format: Ebook
- Item ID: e_3cd6
- Publisher: Packt Publishing