Kategorie
Ebooki
-
Biznes i ekonomia
- Bitcoin
- Bizneswoman
- Coaching
- Controlling
- E-biznes
- Ekonomia
- Finanse
- Giełda i inwestycje
- Kompetencje osobiste
- Komputer w biurze
- Komunikacja i negocjacje
- Mała firma
- Marketing
- Motywacja
- Multimedialne szkolenia
- Nieruchomości
- Perswazja i NLP
- Podatki
- Polityka społeczna
- Poradniki
- Prezentacje
- Przywództwo
- Public Relation
- Raporty, analizy
- Sekret
- Social Media
- Sprzedaż
- Start-up
- Twoja kariera
- Zarządzanie
- Zarządzanie projektami
- Zasoby ludzkie (HR)
-
Dla dzieci
-
Dla młodzieży
-
Edukacja
-
Encyklopedie, słowniki
-
E-prasa
- Architektura i wnętrza
- BHP
- Biznes i Ekonomia
- Dom i ogród
- E-Biznes
- Ekonomia i finanse
- Ezoteryka
- Finanse
- Finanse osobiste
- Firma
- Fotografia
- Informatyka
- Kadry i płace
- Kobieca
- Komputery, Excel
- Księgowość
- Kultura i literatura
- Naukowe i akademickie
- Ochrona środowiska
- Opiniotwórcze
- Oświata
- Podatki
- Podróże
- Psychologia
- Religia
- Rolnictwo
- Rynek książki i prasy
- Transport i Spedycja
- Zdrowie i uroda
-
Historia
-
Informatyka
- Aplikacje biurowe
- Bazy danych
- Bioinformatyka
- Biznes IT
- CAD/CAM
- Digital Lifestyle
- DTP
- Elektronika
- Fotografia cyfrowa
- Grafika komputerowa
- Gry
- Hacking
- Hardware
- IT w ekonomii
- Pakiety naukowe
- Podręczniki szkolne
- Podstawy komputera
- Programowanie
- Programowanie mobilne
- Serwery internetowe
- Sieci komputerowe
- Start-up
- Systemy operacyjne
- Sztuczna inteligencja
- Technologia dla dzieci
- Webmasterstwo
-
Inne
-
Języki obce
-
Kultura i sztuka
-
Lektury szkolne
-
Literatura
- Antologie
- Ballada
- Biografie i autobiografie
- Dla dorosłych
- Dramat
- Dzienniki, pamiętniki, listy
- Epos, epopeja
- Esej
- Fantastyka i science-fiction
- Felietony
- Fikcja
- Humor, satyra
- Inne
- Klasyczna
- Kryminał
- Literatura faktu
- Literatura piękna
- Mity i legendy
- Nobliści
- Nowele
- Obyczajowa
- Okultyzm i magia
- Opowiadania
- Pamiętniki
- Podróże
- Poemat
- Poezja
- Polityka
- Popularnonaukowa
- Powieść
- Powieść historyczna
- Proza
- Przygodowa
- Publicystyka
- Reportaż
- Romans i literatura obyczajowa
- Sensacja
- Thriller, Horror
- Wywiady i wspomnienia
-
Nauki przyrodnicze
-
Nauki społeczne
-
Podręczniki szkolne
-
Popularnonaukowe i akademickie
- Archeologia
- Bibliotekoznawstwo
- Filmoznawstwo
- Filologia
- Filologia polska
- Filozofia
- Finanse i bankowość
- Geografia
- Gospodarka
- Handel. Gospodarka światowa
- Historia i archeologia
- Historia sztuki i architektury
- Kulturoznawstwo
- Lingwistyka
- Literaturoznawstwo
- Logistyka
- Matematyka
- Medycyna
- Nauki humanistyczne
- Pedagogika
- Pomoce naukowe
- Popularnonaukowa
- Pozostałe
- Psychologia
- Socjologia
- Teatrologia
- Teologia
- Teorie i nauki ekonomiczne
- Transport i spedycja
- Wychowanie fizyczne
- Zarządzanie i marketing
-
Poradniki
-
Poradniki do gier
-
Poradniki zawodowe i specjalistyczne
-
Prawo
- BHP
- Historia
- Kodeks drogowy. Prawo jazdy
- Nauki prawne
- Ochrona zdrowia
- Ogólne, kompendium wiedzy
- Podręczniki akademickie
- Pozostałe
- Prawo budowlane i lokalowe
- Prawo cywilne
- Prawo finansowe
- Prawo gospodarcze
- Prawo gospodarcze i handlowe
- Prawo karne
- Prawo karne. Przestępstwa karne. Kryminologia
- Prawo międzynarodowe
- Prawo międzynarodowe i zagraniczne
- Prawo ochrony zdrowia
- Prawo oświatowe
- Prawo podatkowe
- Prawo pracy i ubezpieczeń społecznych
- Prawo publiczne, konstytucyjne i administracyjne
- Prawo rodzinne i opiekuńcze
- Prawo rolne
- Prawo socjalne, prawo pracy
- Prawo Unii Europejskiej
- Przemysł
- Rolne i ochrona środowiska
- Słowniki i encyklopedie
- Zamówienia publiczne
- Zarządzanie
-
Przewodniki i podróże
- Afryka
- Albumy
- Ameryka Południowa
- Ameryka Środkowa i Północna
- Australia, Nowa Zelandia, Oceania
- Austria
- Azja
- Bałkany
- Bliski Wschód
- Bułgaria
- Chiny
- Chorwacja
- Czechy
- Dania
- Egipt
- Estonia
- Europa
- Francja
- Góry
- Grecja
- Hiszpania
- Holandia
- Islandia
- Litwa
- Łotwa
- Mapy, Plany miast, Atlasy
- Miniprzewodniki
- Niemcy
- Norwegia
- Podróże aktywne
- Polska
- Portugalia
- Pozostałe
- Przewodniki po hotelach i restauracjach
- Rosja
- Rumunia
- Słowacja
- Słowenia
- Szwajcaria
- Szwecja
- Świat
- Turcja
- Ukraina
- Węgry
- Wielka Brytania
- Włochy
-
Psychologia
- Filozofie życiowe
- Kompetencje psychospołeczne
- Komunikacja międzyludzka
- Mindfulness
- Ogólne
- Perswazja i NLP
- Psychologia akademicka
- Psychologia duszy i umysłu
- Psychologia pracy
- Relacje i związki
- Rodzicielstwo i psychologia dziecka
- Rozwiązywanie problemów
- Rozwój intelektualny
- Sekret
- Seksualność
- Uwodzenie
- Wygląd i wizerunek
- Życiowe filozofie
-
Religia
-
Sport, fitness, diety
-
Technika i mechanika
Audiobooki
-
Biznes i ekonomia
- Bitcoin
- Bizneswoman
- Coaching
- Controlling
- E-biznes
- Ekonomia
- Finanse
- Giełda i inwestycje
- Kompetencje osobiste
- Komunikacja i negocjacje
- Mała firma
- Marketing
- Motywacja
- Nieruchomości
- Perswazja i NLP
- Podatki
- Polityka społeczna
- Poradniki
- Prezentacje
- Przywództwo
- Public Relation
- Sekret
- Social Media
- Sprzedaż
- Start-up
- Twoja kariera
- Zarządzanie
- Zarządzanie projektami
- Zasoby ludzkie (HR)
-
Dla dzieci
-
Dla młodzieży
-
Edukacja
-
Encyklopedie, słowniki
-
E-prasa
-
Historia
-
Informatyka
-
Inne
-
Języki obce
-
Kultura i sztuka
-
Lektury szkolne
-
Literatura
- Antologie
- Ballada
- Biografie i autobiografie
- Dla dorosłych
- Dramat
- Dzienniki, pamiętniki, listy
- Epos, epopeja
- Esej
- Fantastyka i science-fiction
- Felietony
- Fikcja
- Humor, satyra
- Inne
- Klasyczna
- Kryminał
- Literatura faktu
- Literatura piękna
- Mity i legendy
- Nobliści
- Nowele
- Obyczajowa
- Okultyzm i magia
- Opowiadania
- Pamiętniki
- Podróże
- Poezja
- Polityka
- Popularnonaukowa
- Powieść
- Powieść historyczna
- Proza
- Przygodowa
- Publicystyka
- Reportaż
- Romans i literatura obyczajowa
- Sensacja
- Thriller, Horror
- Wywiady i wspomnienia
-
Nauki przyrodnicze
-
Nauki społeczne
-
Popularnonaukowe i akademickie
-
Poradniki
-
Poradniki zawodowe i specjalistyczne
-
Prawo
-
Przewodniki i podróże
-
Psychologia
- Filozofie życiowe
- Komunikacja międzyludzka
- Mindfulness
- Ogólne
- Perswazja i NLP
- Psychologia akademicka
- Psychologia duszy i umysłu
- Psychologia pracy
- Relacje i związki
- Rodzicielstwo i psychologia dziecka
- Rozwiązywanie problemów
- Rozwój intelektualny
- Sekret
- Seksualność
- Uwodzenie
- Wygląd i wizerunek
- Życiowe filozofie
-
Religia
-
Sport, fitness, diety
-
Technika i mechanika
Kursy video
-
Bazy danych
-
Big Data
-
Biznes, ekonomia i marketing
-
Cyberbezpieczeństwo
-
Data Science
-
DevOps
-
Dla dzieci
-
Elektronika
-
Grafika/Wideo/CAX
-
Gry
-
Microsoft Office
-
Narzędzia programistyczne
-
Programowanie
-
Rozwój osobisty
-
Sieci komputerowe
-
Systemy operacyjne
-
Testowanie oprogramowania
-
Urządzenia mobilne
-
UX/UI
-
Web development
-
Zarządzanie
Podcasty
- Ebooki
- Informatyka
- Programowanie
- Microsoft Visual C++ Windows Applications by Example. Code and explanation for real-world MFC C++ Applications
Szczegóły ebooka
Zaloguj się, jeśli jesteś zainteresowany treścią pozycji.
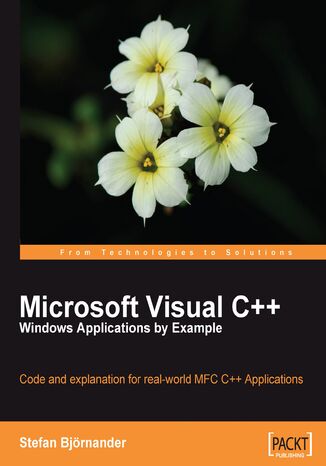
Microsoft Visual C++ Windows Applications by Example. Code and explanation for real-world MFC C++ Applications
Ebook
- Microsoft Visual C++ Windows Applications by Example
- Microsoft Visual C++ Windows Applications by Example
- Credits
- About the Author
- About the Reviewer
- Preface
- What you need for this book
- Who this book is for
- Conventions
- Reader Feedback
- Customer Support
- Downloading the Example Code for the Book
- Errata
- Questions
- 1. Introduction to C++
- The Compiler and the Linker
- The First Program
- Comments
- Types and Variables
- Simple Types
- Variables
- Constants
- Input and Output
- Enumerations
- Arrays
- Pointers and References
- Pointers and Dynamic Memory
- Defining Our Own Types
- The Size and Limits of Types
- Hungarian Notation
- Expressions and Operators
- Arithmetic Operators
- Pointer Arithmetic
- Increment and Decrement
- Relational Operators
- Logical Operators
- Bitwise Operators
- Assignment
- The Condition Operator
- Precedence and Associativity
- Statements
- Selection Statements
- Iteration Statements
- Jump Statements
- Expression Statements
- Functions
- Void Functions
- Local and Global Variables
- Call-by-Value and Call-by-Reference
- Default Parameters
- Overloading
- Static Variables
- Recursion
- Definition and Declaration
- Higher Order Functions
- The main() Function
- The Preprocessor
- The ASCII Table
- Summary
- 2. Object-Oriented Programming in C++
- The Object-Oriented Model
- Classes
- The First Example
- Car.h
- Car.cpp
- Main.cpp
- The Second Example
- BankAccount.h
- BankAccount.cpp
- Main.cpp
- The First Example
- Inheritance
- Person.h
- Person.cpp
- Student.h
- Student.cpp
- Employee.h
- Employee.cpp
- Dynamic Binding
- Main.cpp
- Person.h
- Person.cpp
- Student.h
- Student.cpp
- Employee.h
- Employee.cpp
- Main.cpp
- Arrays of Objects
- Pointers and Linked Lists
- Stacks and Linked Lists
- Cell.h
- Cell.cpp
- Main.cpp
- Stack.h
- Stack.cpp
- Main.cpp
- Stacks and Linked Lists
- Operator Overloading
- Rational.h
- Rational.cpp
- Main.cpp
- Exceptions
- Exception.cpp
- Templates
- TemplateCell.h
- TemplateStack.h
- Main.cpp
- Namespaces
- Streams and File Processing
- TextStream.cpp
- Input.txt
- Output.txt
- BinaryStreams.cpp
- Summary
- 3. Windows Development
- Visual Studio
- The Document/View Model
- The Message System
- The Coordinate System
- The Device Context
- The Registry
- The Cursor
- Serialization
- Summary
- 4. Ring: A Demonstration Example
- The Application Wizard
- Colors and Arrays
- RingDoc.h
- Catching the Mouse
- RingView.cpp
- RingView.cpp
- RingDoc.h
- RingDoc.cpp
- Drawing the Rings
- RingView.cpp
- Setting the Coordinate System and the Scroll Bars
- RingView.cpp
- RingView.cpp
- RingView.cpp
- RingDoc.h
- RingView.cpp
- Catching the Keyboard Input
- RingView.cpp
- Menus, Accelerators, and Toolbars
- RingDoc.h
- RingDoc.cpp
- RingDoc.cpp
- RingDoc.cpp
- The Color Dialog
- RingDoc.cpp
- The Registry
- RingDoc.cpp
- Serialization
- RingDoc.cpp
- RingDoc.cpp
- Summary
- 5. Utility Classes
- The Point, Size, and Rectangle Classes
- The Color Class
- Color.h
- Color.cpp
- The Font Class
- Font.h
- Font.cpp
- The Caret Class
- Caret.h
- Caret.cpp
- The List Class
- List.h
- The Set Class
- Set.h
- Set.cpp
- Set.h
- The Array Class
- Error Handling
- Check.h
- Check.h
- Summary
- 6. The Tetris Application
- The Tetris Files
- The Square Class
- Square.h
- The Color Grid Class
- ColorGrid.h
- ColorGrid.cpp
- The Document Class
- TetrisDoc.h
- TetrisDoc.cpp
- Th e View Class
- TetrisView.h
- TetrisView.cpp
- The Square Class
- The Figure Class
- Figure.h
- Figure.cpp
- The Figure Information
- FigureInfo.cpp
- The Red Figure
- The Brown Figure
- The Turquoise Figure
- The Green Figure
- The Yellow Figure
- The Blue Figure
- The Purple Figure
- Summary
- The Tetris Files
- 7. The Draw Application
- Draw.cpp
- The Resource
- The Class Hierarchy
- The Figure Class
- Figure.h
- Figure.cpp
- The TwoDimensionalFigure Class
- TwoDimensionalFigure.h
- TwoDimensionalFigure.cpp
- The LineFigure Class
- LineFigure.h
- L ineFigure.cpp
- Line Figure.cpp
- LineFigure.cpp
- The ArrowFigure Class
- ArrowFigure.h
- ArrowFigure.cpp
- The RectangleFigure Class
- RectangleFigure.h
- RectangleFigure.cpp
- RectangleFigure.cpp
- The Ell ipseFigure Class
- EllipseFigure.h
- EllipseFigure.cpp
- The TextFigure Class
- TextFigure.h
- TextFigure.cpp
- The FigureFileManager Class
- FigureFileManager.h
- FigureFileManager.cpp
- The Document Class
- DrawDoc.h
- DrawDoc.cpp
- The View Class
- DrawView.h
- CDrawView.cpp
- Summary
- 8. The Calc Application
- Calc.cpp
- The Resource
- Formula Interpretation
- The Tokens
- Token.h
- The Reference Class
- Reference.h
- Reference.cpp
- The ScannerGenerating the List of Tokens
- Scanner.h
- Scanner.cpp
- The ParserGenerating the Syntax Tree
- Parser.h
- Parser.cpp
- The Syntax TreeRepresenting the Formula
- SyntaxTree.h
- SyntaxTree.cpp
- The Tokens
- The Spreadsheet
- The CellHolding Text, Value, or Formula
- Cell.h
- Cell.cpp
- The Cell MatrixManaging Rows and Columns
- CellMatrix.h
- CellMatrix.cpp
- The Target Set Matrix Class
- TSetMatrix.h
- TSetMatrix.cpp
- The CellHolding Text, Value, or Formula
- The Document/View Model
- The Document Class
- CalcDoc.h
- CalcDoc.cpp
- The View Class
- CalcView.h
- CalcView.cpp
- The Document Class
- Summary
- 9. The Word Application
- Word.cpp
- The Resource
- The Line
- Line.h
- Line.cpp
- The Position
- Position.h
- Position.cpp
- The Paragraph
- Paragraph.h
- Paragraph.cpp
- The Line
- The Page
- Page.h
- Page.cpp
- The Document Class
- WordDoc.h
- CWordDoc.cpp
- The View Class
- WordView.h
- CWordView.cpp
- Summary
- Tytuł: Microsoft Visual C++ Windows Applications by Example. Code and explanation for real-world MFC C++ Applications
- Autor: Stefan Bjornander
- Tytuł oryginału: Microsoft Visual C++ Windows Applications by Example. Code and explanation for real-world MFC C++ Applications
- ISBN: 9781847195579, 9781847195579
- Data wydania: 2008-06-26
- Format: Ebook
- Identyfikator pozycji: e_3cmy
- Wydawca: Packt Publishing